qm-dsp
1.8
|
SincWindow.h
Go to the documentation of this file.
SincWindow(int length, double p)
Construct a windower of the given length, containing the values of sinc(x) with x=0 in the middle...
Definition: SincWindow.h:33
Generated by
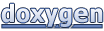