qm-dsp
1.8
|
Decimator carries out a fast downsample by a power-of-two factor. More...
#include <Decimator.h>
Public Member Functions | |
Decimator (int inLength, int decFactor) | |
Construct a Decimator to operate on input blocks of length inLength, with decimation factor decFactor. More... | |
virtual | ~Decimator () |
void | process (const double *src, double *dst) |
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples to dst. More... | |
void | process (const float *src, float *dst) |
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples to dst. More... | |
int | getFactor () const |
void | resetFilter () |
Static Public Member Functions | |
static int | getHighestSupportedFactor () |
Private Member Functions | |
void | deInitialise () |
void | initialise (int inLength, int decFactor) |
void | doAntiAlias (const double *src, double *dst, int length) |
void | doAntiAlias (const float *src, double *dst, int length) |
Private Attributes | |
int | m_inputLength |
int | m_outputLength |
int | m_decFactor |
double | Input |
double | Output |
double | o1 |
double | o2 |
double | o3 |
double | o4 |
double | o5 |
double | o6 |
double | o7 |
double | a [9] |
double | b [9] |
double * | decBuffer |
Detailed Description
Decimator carries out a fast downsample by a power-of-two factor.
Only a limited number of factors are supported, from two to whatever getHighestSupportedFactor() returns. This is much faster than Resampler but has a worse signal-noise ratio.
Definition at line 24 of file Decimator.h.
Constructor & Destructor Documentation
Decimator::Decimator | ( | int | inLength, |
int | decFactor | ||
) |
Construct a Decimator to operate on input blocks of length inLength, with decimation factor decFactor.
inLength should be a multiple of decFactor. Output blocks will be of length inLength / decFactor.
decFactor must be a power of two. The highest supported factor is obtained through getHighestSupportedFactor(); for higher factors, you will need to chain more than one decimator.
Definition at line 24 of file Decimator.cpp.
References initialise(), m_decFactor, m_inputLength, and m_outputLength.
|
virtual |
Definition at line 33 of file Decimator.cpp.
References deInitialise().
Member Function Documentation
void Decimator::process | ( | const double * | src, |
double * | dst | ||
) |
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples to dst.
Note that src and dst may be the same or overlap (an intermediate buffer is used).
Definition at line 195 of file Decimator.cpp.
References decBuffer, doAntiAlias(), m_decFactor, m_inputLength, and m_outputLength.
Referenced by ClusterMeltSegmenter::extractFeaturesConstQ(), ClusterMeltSegmenter::extractFeaturesMFCC(), GetKeyMode::process(), and DownBeat::pushAudioBlock().
void Decimator::process | ( | const float * | src, |
float * | dst | ||
) |
Process inLength samples (as supplied to constructor) from src and write inLength / decFactor samples to dst.
Note that src and dst may be the same or overlap (an intermediate buffer is used).
Definition at line 213 of file Decimator.cpp.
References decBuffer, doAntiAlias(), m_decFactor, m_inputLength, and m_outputLength.
|
inline |
Definition at line 56 of file Decimator.h.
References m_decFactor.
Referenced by ClusterMeltSegmenter::extractFeaturesConstQ(), and ClusterMeltSegmenter::extractFeaturesMFCC().
|
inlinestatic |
Definition at line 57 of file Decimator.h.
References deInitialise(), doAntiAlias(), initialise(), and resetFilter().
Referenced by ClusterMeltSegmenter::initialise(), and DownBeat::makeDecimators().
void Decimator::resetFilter | ( | ) |
Definition at line 148 of file Decimator.cpp.
References Input, o1, o2, o3, o4, o5, o6, o7, and Output.
Referenced by getHighestSupportedFactor(), and initialise().
|
private |
Definition at line 143 of file Decimator.cpp.
References decBuffer.
Referenced by getHighestSupportedFactor(), and ~Decimator().
|
private |
Definition at line 38 of file Decimator.cpp.
References a, b, decBuffer, m_decFactor, m_inputLength, m_outputLength, and resetFilter().
Referenced by Decimator(), and getHighestSupportedFactor().
|
private |
|
private |
Member Data Documentation
|
private |
Definition at line 67 of file Decimator.h.
Referenced by Decimator(), initialise(), and process().
|
private |
Definition at line 68 of file Decimator.h.
Referenced by Decimator(), initialise(), and process().
|
private |
Definition at line 69 of file Decimator.h.
Referenced by Decimator(), getFactor(), initialise(), and process().
|
private |
Definition at line 71 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 72 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 74 of file Decimator.h.
Referenced by doAntiAlias(), and resetFilter().
|
private |
Definition at line 76 of file Decimator.h.
Referenced by doAntiAlias(), and initialise().
|
private |
Definition at line 77 of file Decimator.h.
Referenced by doAntiAlias(), and initialise().
|
private |
Definition at line 79 of file Decimator.h.
Referenced by deInitialise(), initialise(), and process().
The documentation for this class was generated from the following files:
Generated by
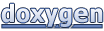