qm-dsp
1.8
|
KaiserWindow.h
Go to the documentation of this file.
Kaiser window: A windower whose bandwidth and sidelobe height (signal-noise ratio) can be specified...
Definition: KaiserWindow.h:25
static KaiserWindow byBandwidth(double attenuation, double bandwidth, double samplerate)
Construct a Kaiser windower with the given attenuation in dB and transition bandwidth in Hz for the g...
Definition: KaiserWindow.h:53
static Parameters parametersForTransitionWidth(double attenuation, double transition)
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition wid...
Definition: KaiserWindow.cpp:19
static Parameters parametersForBandwidth(double attenuation, double bandwidth, double samplerate)
Obtain the parameters necessary for a Kaiser window of the given attenuation in dB and transition ban...
Definition: KaiserWindow.h:72
Definition: KaiserWindow.h:28
static KaiserWindow byTransitionWidth(double attenuation, double transition)
Construct a Kaiser windower with the given attenuation in dB and transition width in samples...
Definition: KaiserWindow.h:43
KaiserWindow(Parameters p)
Construct a Kaiser windower with the given length and beta parameter.
Definition: KaiserWindow.h:37
Generated by
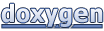