qm-dsp
1.8
|
Resampler.h
Go to the documentation of this file.
Resampler resamples a stream from one integer sample rate to another (arbitrary) rate, using a kaiser-windowed sinc filter.
Definition: Resampler.h:30
int getLatency() const
Return the number of samples of latency at the output due by the filter.
Definition: Resampler.h:68
Definition: Resampler.h:85
int process(const double *src, double *dst, int n)
Read n input samples from src and write resampled data to dst.
Definition: Resampler.cpp:303
Resampler(int sourceRate, int targetRate)
Construct a Resampler to resample from sourceRate to targetRate.
Definition: Resampler.cpp:36
static std::vector< double > resample(int sourceRate, int targetRate, const double *data, int n)
Carry out a one-off resample of a single block of n samples.
Definition: Resampler.cpp:346
Generated by
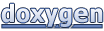