x
|
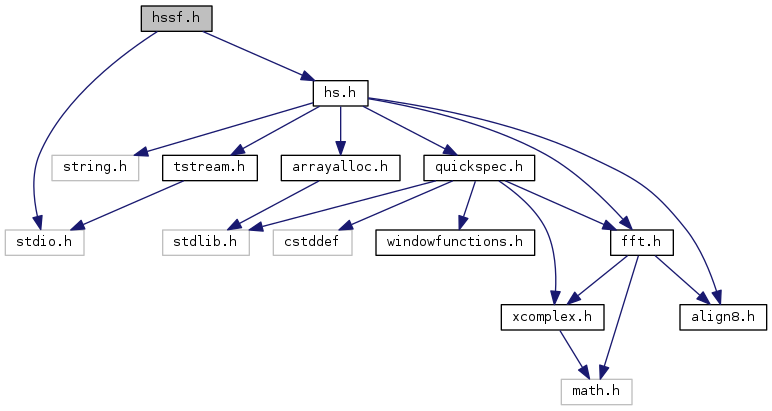
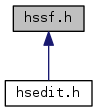
Go to the source code of this file.
Data Structures | |
struct | TSF |
Functions | |
int | Sign (double) |
void | S_F_b (TSF &SF, atom **Partials) |
double | P2_DelFtr (double **d, int L, int K, double **x, double F) |
double | P3_DelSrc (double **d, int L, int M, int K, double **x, double **f, double F) |
int | SF_SV (double **h, int L, int M, int K, double **a, double **f, double F, double theta, double ep, int maxiter) |
double | S_F_SV (int M, atom **Partials, double *logA0C, double *lp, int P, int &K, double **h, double *avgh, double **b, double *avgb, double F=0.005, int FScaleMode=0, double theta=0.5, double Fs=44100) |
void | SF_SV_cf (double *h, double **b, int L, int M, int K, double **a, double **f, double F, double ep, int maxiter) |
double | S_F_SV_cf (int afres, double *LogAF, double *LogAS, int Fr, int M, atom **Partials, double *A0C, double *lp, int P, int &K, double **h, double **b, double F=0.005, int FScaleMode=0, double Fs=44100) |
int | SF_FB (double *hl, int M, int K, double **al, double **fl, double F, int LMode) |
double | S_F_FB (int M, atom **Partials, double *logA0C, double *lp, int P, int &K, double **h, double *avgh, double **b, double *avgb, double F=0.005, int FScaleMode=0, double Fs=44100) |
void | AnalyzeSF_1 (THS &HS, TSF &SF, double sps, double offst) |
void | AnalyzeSF_2 (THS &HS, TSF &SF, double *&cyclefrs, double *&cyclefs, double sps, int *cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0) |
void | AnalyzeSF (THS &HS, TSF &SF, double *&cyclefrs, double *&cyclefs, double sps, double offst, int *cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0) |
void | SynthesizeSF (THS *HS, TSF *SF, double sps) |
Variables | |
const double | Amel =1127.0104803341574386544633680278 |
const bool | useA0 =true |
Detailed Description
- source-filter modeling for harmonic sinusoids
Further reading: Wen X. and M. Sandler, "Source-filter modeling in sinusoid domain," in Proc. AES 126th Convention, Munich, 2009.
Function Documentation
void AnalyzeSF | ( | THS & | HS, |
TSF & | SF, | ||
double *& | cyclefrs, | ||
double *& | cyclefs, | ||
double | sps, | ||
double | offst, | ||
int * | cyclefrcount, | ||
int | SFMode, | ||
double | SFF, | ||
int | SFFScale, | ||
double | SFtheta | ||
) |
function AnalyzeSF: wrapper function.
In: HS: a harmonic sinusoid sps: sampling rate ("samples per second") offst: hop size, the interval between adjacent harmonic atoms of HS SFMode: specifies source-filter estimation criterion, 0=FB (filter bank), 1=SV (slow variation) SFF: filter response sampling interval SFScale: set if filter sampling uses mel scale SFtheta: balancing factor of amplitude and frequency variations, needed for SV approach Out: SF: a TSF object estimated from HS. cyclefrcount: number of cycles cyclefrs[cyclefrcount], cyclefs[cyclefrcount]: average time (in frames) and frequency of each cycle
No return value.
function AnalyzeSF_1: first stage of source-filter model estimation, in which the duration of a HS is segmented into what is equivalent to "cycles" in vibrato analysis.
In: HS: a harmonic sinusoid sps: sampling rate ("samples per second") offst: hop size, the interval between adjacent harmonic atoms of HS Out: SF: a TSF object partially updated, particularly lp[P].
No return value.
void AnalyzeSF_2 | ( | THS & | HS, |
TSF & | SF, | ||
double *& | cyclefrs, | ||
double *& | cyclefs, | ||
double | sps, | ||
int * | cyclefrcount, | ||
int | SFMode, | ||
double | SFF, | ||
int | SFFScale, | ||
double | SFtheta | ||
) |
function AnalyzeSF_2: second stage of source-filter model estimation, in which the HS is demodulated and its source-filter model is estimated.
In: HS: a harmonic sinusoid SF: a TSF object with valid segmentation data (lp[P]) of HS sps: sampling rate ("samples per second") SFMode: specifies source-filter estimation criterion, 0=FB (filter bank), 1=SV (slow variation) SFF: filter response sampling interval SFScale: set if filter sampling uses mel scale SFtheta: balancing factor of amplitude and frequency variations, needed for SV approach Out: SF: the TSF object fully estimated. cyclefrcount: number of cycles cyclefrs[cyclefrcount], cyclefs[cyclefrcount]: average time (in frames) and frequency of each cycle
No return value.
double P2_DelFtr | ( | double ** | d, |
int | L, | ||
int | K, | ||
double ** | x, | ||
double | F | ||
) |
function P2_DelFtr: internal procedure P2 of slow-variation SF estimator. See "further reading", pp.8.
In: x[L][K+2]: filter coefficients F: filter response sampling interval Out: d[L][K+2]: derivative of total filter variation against filter coefficients.
Returns the inner product of x[][] and d[][].
double P3_DelSrc | ( | double ** | d, |
int | L, | ||
int | M, | ||
int | K, | ||
double ** | x, | ||
double ** | f, | ||
double | F | ||
) |
function P3_DelSec: internal procedure P3 of slow-variation SF estimator. See "further reading", pp.9.
In: x[L][K+2]: filter coefficients f[L][M]: partial frequencies F: filter response sampling interval Out: d[L][K+2]: derivative of total source variation against filter coefficients.
Returns the inner product of x[][] and d[][].
double S_F_FB | ( | int | M, |
atom ** | Partials, | ||
double * | logA0C, | ||
double * | lp, | ||
int | P, | ||
int & | K, | ||
double ** | h, | ||
double * | avgh, | ||
double ** | b, | ||
double * | avgb, | ||
double | F, | ||
int | FScaleMode, | ||
double | Fs | ||
) |
function S_F_b: filter-bank method for estimating source-filter model. This is a wrapper function of SF_FB() that transfers and scales values, etc.
In: Partials[M][Fr]: HS partials. Fr is not specified but is assumed no less then lp[P-1]. lp[P]: temporal segmentation points, in frames. logA0C[Fr]: amplitude carrier F: filter response sampling interval FScaleMode: set if mel scale is used as frequency scale Fs: sampling frequency, effective only when FScaleMode is set. Out: K h[L][K+2], aveh[K+2]: filter coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages b[L][M], avgb[M]: source coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages
Returns 0.
double S_F_SV | ( | int | M, |
atom ** | Partials, | ||
double * | logA0C, | ||
double * | lp, | ||
int | P, | ||
int & | K, | ||
double ** | h, | ||
double * | avgh, | ||
double ** | b, | ||
double * | avgb, | ||
double | F, | ||
int | FScaleMode, | ||
double | theta, | ||
double | Fs | ||
) |
function S_F_SV: slow-variation method for estimating source-filter model. This is a wrapper function of SF_SV() that transfers and scales values, etc.
In: Partials[M][Fr]: HS partials. Fr is not specified but is assumed no less then lp[P-1]. lp[P]: temporal segmentation points, in frames. logA0C[Fr]: amplitude carrier theta: balancing factor of amplitude and frequency variations F: filter response sampling interval FScaleMode: set if mel scale is used as frequency scale Fs: sampling frequency, effective only when FScaleMode is set. Out: K h[L][K+2], aveh[K+2]: filter coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages b[L][M], avgb[M]: source coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages Returns 0.
double S_F_SV_cf | ( | int | afres, |
double * | LogAF, | ||
double * | LogAS, | ||
int | Fr, | ||
int | M, | ||
atom ** | Partials, | ||
double * | A0C, | ||
double * | lp, | ||
int | P, | ||
int & | K, | ||
double ** | h, | ||
double ** | b, | ||
double | F, | ||
int | FScaleMode, | ||
double | Fs | ||
) |
function S_F_SV_cf: slow-variation method for estimating source-(constant)filter model. This is a wrapper function of SF_SV_cf() that transfers and scales values, as well as converting results to source filter format used by the vibrato class TVo.
In: Partials[M][Fr]: HS partials. Fr is not specified but is assumed no less then lp[P-1]. lp[P]: temporal segmentation points, in frames. A0C[Fr]: amplitude carrier (linear, not dB) F: filter response sampling interval FScaleMode: set if mel scale is used as frequency scale Fs: sampling frequency, effective only when FScaleMode is set. Out: K h[L][K+2], aveh[K+2]: filter coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages b[L][M], avgb[M]: source coefficients for L frames from ceil(lp[0]) to ceil(lp[P-1]), and their averages LogAF[afres/2]: TVo-format filter response LogAS[M]: TVo-format source response
Returns 0.
int SF_FB | ( | double * | hl, |
int | M, | ||
int | K, | ||
double ** | al, | ||
double ** | fl, | ||
double | F, | ||
int | LMode | ||
) |
function SF_FB: computes filter coefficients with filter-bank method: single frame. See "further reading", pp.12, P5.
In: al[][M], fl[][M]: partial amplitudes and frequencies, al[0] and fl[0] are of current frame F: filter response sampling interval LMode: 1: current frame is the first frame; -1: the last frame; 0: neither first nor last frame Out: hl[K+2]: filter coefficients integrated at current frame
Returns K.
int SF_SV | ( | double ** | h, |
int | L, | ||
int | M, | ||
int | K, | ||
double ** | a, | ||
double ** | f, | ||
double | F, | ||
double | theta, | ||
double | ep, | ||
int | maxiter | ||
) |
function SF_SV: CG method for estimation source-filter model using slow-variation criterion. See "further reading", pp.9, boxed algorithm P4.
In: a[L][M], f[L][M]: partial amplitudes and frequencies F: filter response sampling interval theta: balancing factor of amplitude and frequency variations ep: convergence test threshold maxiter: maximal number of iterates Out: h[L][K+2]: filter coefficients
Returns K.
void SF_SV_cf | ( | double * | h, |
double ** | b, | ||
int | L, | ||
int | M, | ||
int | K, | ||
double ** | a, | ||
double ** | f, | ||
double | F, | ||
double | ep, | ||
int | maxiter | ||
) |
function SF_SV_cf: CG method for estimation source-(constant)filter model by slow-variation criterion.
In: a[L][M], f[L][M]: partial amplitudes and frequencies F: filter response sampling interval ep: convergence test threshold maxiter: maximal number of iterates Out: h[K+2]: filter coefficients b[L][M]: source coefficients
No reutrn value.
int Sign | ( | double | x | ) |
function Sign: sign function
In: x: a floating-point number
Returns 0 if x=0, 1 if x>0, -1 if x<0.
Generated on Mon Feb 17 2025 07:04:33 for x by
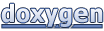