x
|
hssf.h
Go to the documentation of this file.
29 const bool useA0=true; //if true, use A0D+A0C instead of A0C in S-F decomposition as pre-normalizer
114 int SF_SV(double** h, int L, int M, int K, double** a, double** f, double F, double theta, double ep, int maxiter);
115 double S_F_SV(int M, atom** Partials, double* logA0C, double* lp, int P, int& K, double** h, double* avgh, double** b, double* avgb, double F=0.005, int FScaleMode=0, double theta=0.5, double Fs=44100);
116 void SF_SV_cf(double* h, double** b, int L, int M, int K, double** a, double** f, double F, double ep, int maxiter);
117 double S_F_SV_cf(int afres, double* LogAF, double* LogAS, int Fr, int M, atom** Partials, double* A0C, double* lp, int P, int& K, double** h, double** b, double F=0.005, int FScaleMode=0, double Fs=44100);
121 double S_F_FB(int M, atom** Partials, double* logA0C, double* lp, int P, int& K, double** h, double* avgh, double** b, double* avgb, double F=0.005, int FScaleMode=0, double Fs=44100);
125 void AnalyzeSF_2(THS& HS, TSF& SF, double*& cyclefrs, double*& cyclefs, double sps, int* cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0);
126 void AnalyzeSF(THS& HS, TSF& SF, double*& cyclefrs, double*& cyclefs, double sps, double offst, int* cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0);
double S_F_FB(int M, atom **Partials, double *logA0C, double *lp, int P, int &K, double **h, double *avgh, double **b, double *avgb, double F=0.005, int FScaleMode=0, double Fs=44100)
Definition: hssf.cpp:670
int SF_SV(double **h, int L, int M, int K, double **a, double **f, double F, double theta, double ep, int maxiter)
Definition: hssf.cpp:1002
Definition: hs.h:49
double P3_DelSrc(double **d, int L, int M, int K, double **x, double **f, double F)
Definition: hssf.cpp:574
void AnalyzeSF(THS &HS, TSF &SF, double *&cyclefrs, double *&cyclefs, double sps, double offst, int *cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0)
Definition: hssf.cpp:264
double S_F_SV(int M, atom **Partials, double *logA0C, double *lp, int P, int &K, double **h, double *avgh, double **b, double *avgb, double F=0.005, int FScaleMode=0, double theta=0.5, double Fs=44100)
Definition: hssf.cpp:733
void AnalyzeSF_2(THS &HS, TSF &SF, double *&cyclefrs, double *&cyclefs, double sps, int *cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0)
Definition: hssf.cpp:351
void SF_SV_cf(double *h, double **b, int L, int M, int K, double **a, double **f, double F, double ep, int maxiter)
Definition: hssf.cpp:1104
double S_F_SV_cf(int afres, double *LogAF, double *LogAS, int Fr, int M, atom **Partials, double *A0C, double *lp, int P, int &K, double **h, double **b, double F=0.005, int FScaleMode=0, double Fs=44100)
Definition: hssf.cpp:794
Definition: hs.h:147
Definition: hssf.h:40
int SF_FB(double *hl, int M, int K, double **al, double **fl, double F, int LMode)
Definition: hssf.cpp:875
Generated on Thu May 2 2024 07:03:53 for x by
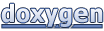