svcore
1.9
|
ViewManagerBase.h
Go to the documentation of this file.
virtual void setAudioRecordTarget(AudioRecordTarget *target)=0
void playSoloModeChanged()
Emitted when the play solo mode has been changed.
virtual sv_frame_t constrainFrameToSelection(sv_frame_t frame) const =0
A selection object simply represents a range in time, via start and end frame.
Definition: Selection.h:40
void playLoopModeChanged()
Emitted when the play loop mode has been changed.
virtual const MultiSelection::SelectionList & getSelections() const =0
virtual sv_frame_t alignReferenceToPlaybackFrame(sv_frame_t) const =0
void alignModeChanged()
Emitted when the alignment mode has been changed.
Definition: Selection.h:63
virtual const MultiSelection & getSelection() const =0
virtual void setAudioPlaySource(AudioPlaySource *source)=0
void playSelectionModeChanged()
Emitted when the play selection mode has been changed.
virtual sv_frame_t alignPlaybackFrameToReference(sv_frame_t) const =0
virtual bool getPlaySoloMode() const =0
virtual bool getAlignMode() const =0
virtual bool getPlayLoopMode() const =0
virtual bool getPlaySelectionMode() const =0
virtual Selection getContainingSelection(sv_frame_t frame, bool defaultToFollowing) const =0
Generated by
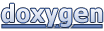