svcore
1.9
|
PowerOfTwoZoomConstraint.cpp
Go to the documentation of this file.
virtual int getNearestBlockSize(int requested, RoundingDirection dir=RoundNearest) const
Definition: PowerOfTwoZoomConstraint.cpp:47
virtual ZoomLevel getMaxZoomLevel() const
Return the maximum zoom level within range for this constraint.
Definition: ZoomConstraint.h:81
virtual ZoomLevel getMinZoomLevel() const
Return the minimum zoom level within range for this constraint.
Definition: ZoomConstraint.h:71
Definition: ZoomConstraint.h:40
virtual ZoomLevel getNearestZoomLevel(ZoomLevel requested, RoundingDirection dir=RoundNearest) const override
Given an "ideal" zoom level (frames per pixel or pixels per frame) for a given zoom level...
Definition: PowerOfTwoZoomConstraint.cpp:19
Definition: ZoomConstraint.h:38
Definition: ZoomConstraint.h:39
Definition: ZoomLevel.h:29
Generated by
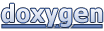