svcore
1.9
|
PlaylistFileReader.h
Go to the documentation of this file.
virtual ~PlaylistFileReader()
Definition: PlaylistFileReader.cpp:50
static void getSupportedExtensions(std::set< QString > &extensions)
Definition: PlaylistFileReader.cpp:153
PlaylistFileReader(QString path)
Definition: PlaylistFileReader.cpp:26
static bool isSupported(FileSource source)
Definition: PlaylistFileReader.cpp:159
FileSource is a class used to refer to the contents of a file that may be either local or at a remote...
Definition: FileSource.h:59
Definition: PlaylistFileReader.h:28
Generated by
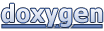