svcore
1.9
|
BasicCompressedDenseThreeDimensionalModel.h
Go to the documentation of this file.
QString m_binValueUnit
Definition: BasicCompressedDenseThreeDimensionalModel.h:215
QVector< QVector< QString > > toStringExportRows(DataExportOptions options, sv_frame_t startFrame, sv_frame_t duration) const override
Emit events starting within the given range as string rows ready for conversion to an e...
virtual void setBinValues(std::vector< float > values)
Set the values of all bins (separate from their labels).
sv_samplerate_t getSampleRate() const override
Return the frame rate in frames per second.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:66
bool isOK() const override
Return true if the model was constructed successfully.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:53
int m_completion
Definition: BasicCompressedDenseThreeDimensionalModel.h:227
sv_frame_t m_sinceLastNotifyMin
Definition: BasicCompressedDenseThreeDimensionalModel.h:225
sv_frame_t m_startFrame
Definition: BasicCompressedDenseThreeDimensionalModel.h:217
void toXml(QTextStream &out, QString indent="", QString extraAttributes="") const override
Stream this exportable object out to XML on a text stream.
bool m_notifyOnAdd
Definition: BasicCompressedDenseThreeDimensionalModel.h:224
float getMaximumLevel() const override
Return the maximum value of the value in each bin.
std::vector< float > m_binValues
Definition: BasicCompressedDenseThreeDimensionalModel.h:214
virtual void setResolution(int sz)
Set the number of sample frames covered by each set of bins.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:96
virtual void setBinValueUnit(QString unit)
Set the name of the unit of the values return from getBinValue() if any.
virtual void setBinName(int n, QString)
Set the name of bin n.
virtual void setStartFrame(sv_frame_t)
Set the frame offset of the first column.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:78
virtual void setBinNames(std::vector< QString > names)
Set the names of all bins.
QVector< QString > getStringExportHeaders(DataExportOptions options) const override
Return a label for each column that would be written by toStringExportRows.
void truncateAndStore(int index, const Column &values)
float m_minimum
Definition: BasicCompressedDenseThreeDimensionalModel.h:221
QReadWriteLock m_lock
Definition: BasicCompressedDenseThreeDimensionalModel.h:229
float getMinimumLevel() const override
Return the minimum value of the value in each bin.
float getValueAt(int x, int n) const override
Get a single value, from the n'th bin of the given column.
int m_yBinCount
Definition: BasicCompressedDenseThreeDimensionalModel.h:220
QString getBinName(int n) const override
Return the name of bin n.
float m_maximum
Definition: BasicCompressedDenseThreeDimensionalModel.h:222
int getResolution() const override
Return the number of sample frames covered by each set of bins.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:90
bool hasBinValues() const override
Return true if the bins have values as well as names.
bool shouldUseLogValueScale() const override
Return true if the distribution of values in the bins is such as to suggest a log scale (mapping to c...
sv_frame_t getTrueEndFrame() const override
Return the audio frame at the end of the model.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:84
sv_frame_t m_sinceLastNotifyMax
Definition: BasicCompressedDenseThreeDimensionalModel.h:226
Column expandAndRetrieve(int index) const
virtual void setMinimumLevel(float sz)
Set the minimum value of the value in a bin.
void setCompletion(int completion, bool update=true)
ValueMatrix m_data
Definition: BasicCompressedDenseThreeDimensionalModel.h:198
int getWidth() const override
Return the number of columns.
Column getColumn(int x) const override
Get the set of bin values at the given column.
std::vector< QString > m_binNames
Definition: BasicCompressedDenseThreeDimensionalModel.h:213
virtual void setHeight(int sz)
Set the number of bins in each column.
virtual void setMaximumLevel(float sz)
Set the maximum value of the value in a bin.
QString getBinValueUnit() const override
Obtain the name of the unit of the values returned from getBinValue(), if any.
bool m_haveExtents
Definition: BasicCompressedDenseThreeDimensionalModel.h:223
Column rightHeight(const Column &c) const
int m_resolution
Definition: BasicCompressedDenseThreeDimensionalModel.h:219
QString getTypeName() const override
Return the type of the model.
Definition: BasicCompressedDenseThreeDimensionalModel.h:182
virtual void setColumn(int x, const Column &values)
Set the entire set of bin values at the given column.
sv_frame_t getStartFrame() const override
Return the first audio frame spanned by the model.
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:72
float getBinValue(int n) const override
Return the value of bin n, if any.
sv_samplerate_t m_sampleRate
Definition: BasicCompressedDenseThreeDimensionalModel.h:218
std::vector< Column > ValueMatrix
Definition: BasicCompressedDenseThreeDimensionalModel.h:197
ColumnOp::Column Column
Definition: DenseThreeDimensionalModel.h:59
int getCompletion() const override
Return an estimated percentage value showing how far through any background operation used to calcula...
BasicCompressedDenseThreeDimensionalModel(sv_samplerate_t sampleRate, int resolution, int height, bool notifyOnAdd=true)
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:34
bool isReady(int *completion=0) const override
Return true if the model has finished loading or calculating all its data, for a model that is capabl...
Definition: BasicCompressedDenseThreeDimensionalModel.cpp:59
std::vector< signed char > m_trunc
Definition: BasicCompressedDenseThreeDimensionalModel.h:208
int getHeight() const override
Return the number of bins in each column.
Generated by
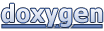