qm-dsp
1.8
|
#include <DCT.h>
Public Member Functions | |
DCT (int n) | |
Construct a DCT object to calculate the Discrete Cosine Transform given input of size n samples. More... | |
~DCT () | |
void | forward (const double *in, double *out) |
Carry out a type-II DCT of size n, where n is the value provided to the constructor above. More... | |
void | forwardUnitary (const double *in, double *out) |
Carry out a type-II unitary DCT of size n, where n is the value provided to the constructor above. More... | |
void | inverse (const double *in, double *out) |
Carry out a type-III (inverse) DCT of size n, where n is the value provided to the constructor above. More... | |
void | inverseUnitary (const double *in, double *out) |
Carry out a type-III (inverse) unitary DCT of size n, where n is the value provided to the constructor above. More... | |
Private Attributes | |
int | m_n |
double | m_scale |
std::vector< double > | m_scaled |
std::vector< double > | m_time2 |
std::vector< double > | m_freq2r |
std::vector< double > | m_freq2i |
FFTReal | m_fft |
Detailed Description
Constructor & Destructor Documentation
DCT::DCT | ( | int | n | ) |
Member Function Documentation
void DCT::forward | ( | const double * | in, |
double * | out | ||
) |
Carry out a type-II DCT of size n, where n is the value provided to the constructor above.
The in and out pointers must point to (enough space for) n values.
Definition at line 36 of file DCT.cpp.
References FFTReal::forward(), m_fft, m_freq2i, m_freq2r, m_n, and m_time2.
Referenced by forwardUnitary().
void DCT::forwardUnitary | ( | const double * | in, |
double * | out | ||
) |
Carry out a type-II unitary DCT of size n, where n is the value provided to the constructor above.
This is a scaled version of the type-II DCT corresponding to a transform with an orthogonal matrix. This is the transform implemented by the dct() function in MATLAB.
The in and out pointers must point to (enough space for) n values.
void DCT::inverse | ( | const double * | in, |
double * | out | ||
) |
Carry out a type-III (inverse) DCT of size n, where n is the value provided to the constructor above.
The in and out pointers must point to (enough space for) n values.
Definition at line 61 of file DCT.cpp.
References FFTReal::inverse(), m_fft, m_freq2i, m_freq2r, m_n, and m_time2.
Referenced by inverseUnitary().
void DCT::inverseUnitary | ( | const double * | in, |
double * | out | ||
) |
Carry out a type-III (inverse) unitary DCT of size n, where n is the value provided to the constructor above.
This is the inverse of forwardUnitary().
The in and out pointers must point to (enough space for) n values.
Member Data Documentation
|
private |
Definition at line 76 of file DCT.h.
Referenced by DCT(), forward(), forwardUnitary(), inverse(), and inverseUnitary().
|
private |
Definition at line 77 of file DCT.h.
Referenced by DCT(), forwardUnitary(), and inverseUnitary().
|
private |
Definition at line 78 of file DCT.h.
Referenced by inverseUnitary().
|
private |
|
private |
|
private |
|
private |
The documentation for this class was generated from the following files:
Generated by
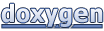