qm-dsp
1.8
|
DCT.cpp
Go to the documentation of this file.
void forward(const double *in, double *out)
Carry out a type-II DCT of size n, where n is the value provided to the constructor above...
Definition: DCT.cpp:36
void inverseUnitary(const double *in, double *out)
Carry out a type-III (inverse) unitary DCT of size n, where n is the value provided to the constructo...
Definition: DCT.cpp:83
void inverse(const double *realIn, const double *imagIn, double *realOut)
Carry out an inverse real transform (i.e.
Definition: FFT.cpp:196
void forward(const double *realIn, double *realOut, double *imagOut)
Carry out a forward real-to-complex transform of size nsamples, where nsamples is the value provided ...
Definition: FFT.cpp:184
DCT(int n)
Construct a DCT object to calculate the Discrete Cosine Transform given input of size n samples...
Definition: DCT.cpp:20
void forwardUnitary(const double *in, double *out)
Carry out a type-II unitary DCT of size n, where n is the value provided to the constructor above...
Definition: DCT.cpp:51
void inverse(const double *in, double *out)
Carry out a type-III (inverse) DCT of size n, where n is the value provided to the constructor above...
Definition: DCT.cpp:61
Generated by
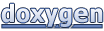