qm-dsp
1.8
|
PeakPicking.h
Go to the documentation of this file.
Definition: PeakPicking.h:58
Definition: DFProcess.h:57
Definition: PeakPicking.h:44
Definition: PeakPicking.h:32
Definition: DFProcess.h:31
Definition: PeakPicking.h:86
Generated by
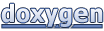