FFmpeg
|
Go to the source code of this file.
Macros | |
#define | MOVNTQ "movq" |
#define | SFENCE " # nop" |
#define | REG_BLUE "0" |
#define | REG_RED "1" |
#define | REG_GREEN "2" |
#define | REG_ALPHA "3" |
#define | YUV2RGB_LOOP(depth) |
#define | YUV2RGB_INITIAL_LOAD |
#define | YUV2RGB |
#define | RGB_PACK_INTERLEAVE |
#define | YUV2RGB_ENDLOOP(depth) |
#define | YUV2RGB_OPERANDS |
#define | YUV2RGB_OPERANDS_ALPHA |
#define | YUV2RGB_ENDFUNC |
#define | IF0(x) |
#define | IF1(x) x |
#define | RGB_PACK16(gmask, is15) |
#define | DITHER_RGB |
#define | RGB_PACK24(blue, red) |
#define | RGB_PACK24_B |
#define | SET_EMPTY_ALPHA "pcmpeqd %%mm"REG_ALPHA", %%mm"REG_ALPHA"\n\t" /* set alpha to 0xFF */ \ |
#define | LOAD_ALPHA "movq (%6, %0, 2), %%mm"REG_ALPHA"\n\t" \ |
#define | RGB_PACK32(red, green, blue, alpha) |
Functions | |
static int RENAME() | yuv420_rgb15 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
static int RENAME() | yuv420_rgb16 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
static int RENAME() | yuv420_rgb24 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
static int RENAME() | yuv420_bgr24 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
static int RENAME() | yuv420_rgb32 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
static int RENAME() | yuv420_bgr32 (SwsContext *c, const uint8_t *src[], int srcStride[], int srcSliceY, int srcSliceH, uint8_t *dst[], int dstStride[]) |
Macro Definition Documentation
#define DITHER_RGB |
Definition at line 179 of file yuv2rgb_template.c.
Referenced by yuv420_rgb15(), and yuv420_rgb16().
#define IF0 | ( | x | ) |
Definition at line 158 of file yuv2rgb_template.c.
Definition at line 159 of file yuv2rgb_template.c.
#define LOAD_ALPHA "movq (%6, %0, 2), %%mm"REG_ALPHA"\n\t" \ |
Definition at line 343 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
#define MOVNTQ "movq" |
Definition at line 32 of file yuv2rgb_template.c.
#define REG_ALPHA "3" |
Definition at line 39 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
#define REG_BLUE "0" |
Definition at line 36 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb24(), and yuv420_rgb32().
#define REG_GREEN "2" |
Definition at line 38 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
#define REG_RED "1" |
Definition at line 37 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb24(), and yuv420_rgb32().
#define RGB_PACK16 | ( | gmask, | |
is15 | |||
) |
Definition at line 161 of file yuv2rgb_template.c.
Referenced by yuv420_rgb15(), and yuv420_rgb16().
#define RGB_PACK24 | ( | blue, | |
red | |||
) |
Definition at line 242 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), and yuv420_rgb24().
#define RGB_PACK24_B |
Definition at line 287 of file yuv2rgb_template.c.
#define RGB_PACK32 | ( | red, | |
green, | |||
blue, | |||
alpha | |||
) |
Definition at line 346 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
#define RGB_PACK_INTERLEAVE |
Definition at line 118 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), and yuv420_rgb32().
Definition at line 340 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
#define SFENCE " # nop" |
Definition at line 33 of file yuv2rgb_template.c.
#define YUV2RGB |
Definition at line 79 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_ENDFUNC |
Definition at line 153 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_ENDLOOP | ( | depth | ) |
Definition at line 129 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_INITIAL_LOAD |
Definition at line 56 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_LOOP | ( | depth | ) |
Definition at line 41 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_OPERANDS |
Definition at line 137 of file yuv2rgb_template.c.
Referenced by yuv420_bgr24(), yuv420_bgr32(), yuv420_rgb15(), yuv420_rgb16(), yuv420_rgb24(), and yuv420_rgb32().
#define YUV2RGB_OPERANDS_ALPHA |
Definition at line 145 of file yuv2rgb_template.c.
Referenced by yuv420_bgr32(), and yuv420_rgb32().
Function Documentation
|
inlinestatic |
Definition at line 321 of file yuv2rgb_template.c.
|
inlinestatic |
Definition at line 408 of file yuv2rgb_template.c.
|
inlinestatic |
Definition at line 185 of file yuv2rgb_template.c.
|
inlinestatic |
Definition at line 213 of file yuv2rgb_template.c.
|
inlinestatic |
Definition at line 303 of file yuv2rgb_template.c.
|
inlinestatic |
Definition at line 365 of file yuv2rgb_template.c.
Generated on Fri Dec 20 2024 06:56:17 for FFmpeg by
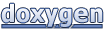