FFmpeg
|
VC-1 and WMV3 decoder. More...
#include "libavutil/avassert.h"
#include "libavutil/common.h"
#include "h264chroma.h"
#include "rnd_avg.h"
#include "vc1dsp.h"
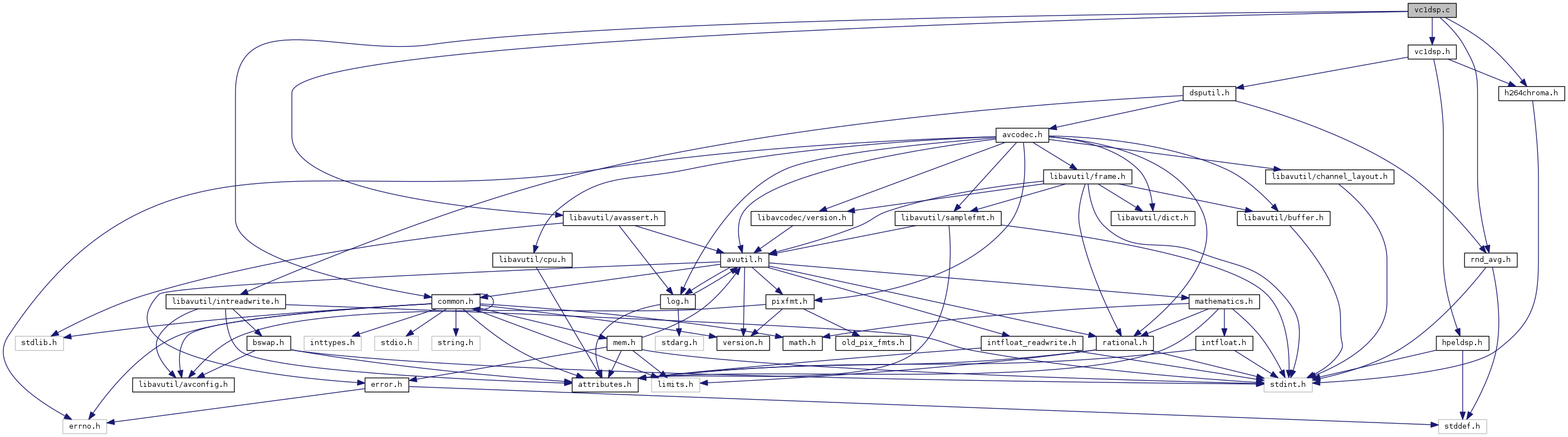
Go to the source code of this file.
Macros | |
#define | VC1_MSPEL_FILTER_16B(DIR, TYPE) |
Filter in case of 2 filters. More... | |
#define | VC1_MSPEL_MC(OP, OP4, OPNAME) |
Function used to do motion compensation with bicubic interpolation. More... | |
#define | op_put(a, b) a = av_clip_uint8(b) |
#define | op_avg(a, b) a = (a + av_clip_uint8(b) + 1) >> 1 |
#define | op4_avg(a, b) a = rnd_avg32(a, b) |
#define | op4_put(a, b) a = b |
#define | PUT_VC1_MSPEL(a, b) |
#define | avg2(a, b) ((a+b+1)>>1) |
Functions | |
static void | vc1_v_overlap_c (uint8_t *src, int stride) |
Apply overlap transform to horizontal edge. More... | |
static void | vc1_h_overlap_c (uint8_t *src, int stride) |
Apply overlap transform to vertical edge. More... | |
static void | vc1_v_s_overlap_c (int16_t *top, int16_t *bottom) |
static void | vc1_h_s_overlap_c (int16_t *left, int16_t *right) |
static av_always_inline int | vc1_filter_line (uint8_t *src, int stride, int pq) |
VC-1 in-loop deblocking filter for one line. More... | |
static void | vc1_loop_filter (uint8_t *src, int step, int stride, int len, int pq) |
VC-1 in-loop deblocking filter. More... | |
static void | vc1_v_loop_filter4_c (uint8_t *src, int stride, int pq) |
static void | vc1_h_loop_filter4_c (uint8_t *src, int stride, int pq) |
static void | vc1_v_loop_filter8_c (uint8_t *src, int stride, int pq) |
static void | vc1_h_loop_filter8_c (uint8_t *src, int stride, int pq) |
static void | vc1_v_loop_filter16_c (uint8_t *src, int stride, int pq) |
static void | vc1_h_loop_filter16_c (uint8_t *src, int stride, int pq) |
static void | vc1_inv_trans_8x8_dc_c (uint8_t *dest, int linesize, int16_t *block) |
Do inverse transform on 8x8 block. More... | |
static void | vc1_inv_trans_8x8_c (int16_t block[64]) |
static void | vc1_inv_trans_8x4_dc_c (uint8_t *dest, int linesize, int16_t *block) |
Do inverse transform on 8x4 part of block. More... | |
static void | vc1_inv_trans_8x4_c (uint8_t *dest, int linesize, int16_t *block) |
static void | vc1_inv_trans_4x8_dc_c (uint8_t *dest, int linesize, int16_t *block) |
Do inverse transform on 4x8 parts of block. More... | |
static void | vc1_inv_trans_4x8_c (uint8_t *dest, int linesize, int16_t *block) |
static void | vc1_inv_trans_4x4_dc_c (uint8_t *dest, int linesize, int16_t *block) |
Do inverse transform on 4x4 part of block. More... | |
static void | vc1_inv_trans_4x4_c (uint8_t *dest, int linesize, int16_t *block) |
static av_always_inline int | vc1_mspel_filter (const uint8_t *src, int stride, int mode, int r) |
Filter used to interpolate fractional pel values. More... | |
static void | put_no_rnd_vc1_chroma_mc8_c (uint8_t *dst, uint8_t *src, int stride, int h, int x, int y) |
static void | put_no_rnd_vc1_chroma_mc4_c (uint8_t *dst, uint8_t *src, int stride, int h, int x, int y) |
static void | avg_no_rnd_vc1_chroma_mc8_c (uint8_t *dst, uint8_t *src, int stride, int h, int x, int y) |
av_cold void | ff_vc1dsp_init (VC1DSPContext *dsp) |
Detailed Description
VC-1 and WMV3 decoder.
Definition in file vc1dsp.c.
Macro Definition Documentation
Definition at line 719 of file vc1dsp.c.
Referenced by avg_no_rnd_vc1_chroma_mc8_c().
#define VC1_MSPEL_FILTER_16B | ( | DIR, | |
TYPE | |||
) |
Filter in case of 2 filters.
#define VC1_MSPEL_MC | ( | OP, | |
OP4, | |||
OPNAME | |||
) |
Function Documentation
|
static |
Definition at line 720 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
av_cold void ff_vc1dsp_init | ( | VC1DSPContext * | dsp | ) |
Definition at line 804 of file vc1dsp.c.
Referenced by vc1_decode_init(), and wmv9_init().
|
static |
Definition at line 699 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
|
static |
Definition at line 675 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
|
static |
VC-1 in-loop deblocking filter for one line.
- Parameters
-
src source block type stride block stride pq block quantizer
- Returns
- whether other 3 pairs should be filtered or not
- See also
- 8.6
Definition at line 145 of file vc1dsp.c.
Referenced by vc1_loop_filter().
Definition at line 228 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 208 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 218 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Apply overlap transform to vertical edge.
Definition at line 62 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
|
static |
Definition at line 111 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 486 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Do inverse transform on 4x4 part of block.
Definition at line 471 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 415 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Do inverse transform on 4x8 parts of block.
Definition at line 400 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 344 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Do inverse transform on 8x4 part of block.
Definition at line 325 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
|
static |
Definition at line 254 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Do inverse transform on 8x8 block.
Definition at line 235 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
VC-1 in-loop deblocking filter.
- Parameters
-
src source block type step distance between horizontally adjacent elements stride distance between vertically adjacent elements len edge length to filter (4 or 8 pixels) pq block quantizer
- See also
- 8.6
Definition at line 187 of file vc1dsp.c.
Referenced by vc1_h_loop_filter16_c(), vc1_h_loop_filter4_c(), vc1_h_loop_filter8_c(), vc1_v_loop_filter16_c(), vc1_v_loop_filter4_c(), and vc1_v_loop_filter8_c().
|
static |
Definition at line 223 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 203 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Definition at line 213 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Apply overlap transform to horizontal edge.
Definition at line 37 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
|
static |
Definition at line 85 of file vc1dsp.c.
Referenced by ff_vc1dsp_init().
Generated on Fri Aug 1 2025 06:53:55 for FFmpeg by
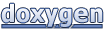