FFmpeg
|
soxr_resample.c
Go to the documentation of this file.
32 static struct ResampleContext *create(struct ResampleContext *c, int out_rate, int in_rate, int filter_size, int phase_shift, int linear,
33 double cutoff, enum AVSampleFormat format, enum SwrFilterType filter_type, int kaiser_beta, double precision, int cheby){
48 soxr_quality_spec_t q_spec = soxr_quality_spec((int)((precision-2)/4), (SOXR_HI_PREC_CLOCK|SOXR_ROLLOFF_NONE)*!!cheby);
static struct ResampleContext * create(struct ResampleContext *c, int out_rate, int in_rate, int filter_size, int phase_shift, int linear, double cutoff, enum AVSampleFormat format, enum SwrFilterType filter_type, int kaiser_beta, double precision, int cheby)
Definition: soxr_resample.c:32
Audio buffer used for intermediate storage between conversion phases.
Definition: oss_audio.c:46
Definition: af_resample.c:39
static int process(struct ResampleContext *c, AudioData *dst, int dst_size, AudioData *src, int src_size, int *consumed)
Definition: soxr_resample.c:74
Definition: swresample_internal.h:159
enum AVResampleFilterType filter_type
Definition: libavresample/resample.c:43
Definition: swresample_internal.h:69
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
#define type
Generated on Wed Apr 16 2025 06:53:20 for FFmpeg by
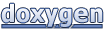