FFmpeg
|
rv34dsp.h
Go to the documentation of this file.
rv40_loop_filter_strength_func rv40_loop_filter_strength[2]
Definition: rv34dsp.h:74
void(* rv40_weight_func)(uint8_t *dst, uint8_t *src1, uint8_t *src2, int w1, int w2, ptrdiff_t stride)
Definition: rv34dsp.h:33
rv40_weak_loop_filter_func rv40_weak_loop_filter[2]
Definition: rv34dsp.h:72
void(* rv34_idct_dc_add_func)(uint8_t *dst, ptrdiff_t stride, int dc)
Definition: rv34dsp.h:41
rv40_weight_func rv40_weight_pixels_tab[2][2]
Biweight functions, first dimension is transform size (16/8), second is whether the weight is prescal...
Definition: rv34dsp.h:67
int(* rv40_loop_filter_strength_func)(uint8_t *src, ptrdiff_t stride, int beta, int beta2, int edge, int *p1, int *q1)
Definition: rv34dsp.h:53
void(* qpel_mc_func)(uint8_t *dst, uint8_t *src, ptrdiff_t stride)
Definition: dsputil.h:84
struct RV34DSPContext RV34DSPContext
rv34_inv_transform_func rv34_inv_transform_dc
Definition: rv34dsp.h:69
Definition: rv34dsp.h:57
void(* h264_chroma_mc_func)(uint8_t *dst, uint8_t *src, int srcStride, int h, int x, int y)
Definition: h264chroma.h:24
void(* rv34_idct_add_func)(uint8_t *dst, ptrdiff_t stride, int16_t *block)
Definition: rv34dsp.h:40
FIXME Range Coding of cr are mx and my are Motion Vector top and top right vectors is used as motion vector prediction the used motion vector is the sum of the predictor and(mvx_diff, mvy_diff)*mv_scale Intra DC Predicton block[y][x] dc[1]
Definition: snow.txt:392
void(* rv40_strong_loop_filter_func)(uint8_t *src, ptrdiff_t stride, int alpha, int lims, int dmode, int chroma)
Definition: rv34dsp.h:49
rv40_strong_loop_filter_func rv40_strong_loop_filter[2]
Definition: rv34dsp.h:73
void(* rv40_weak_loop_filter_func)(uint8_t *src, ptrdiff_t stride, int filter_p1, int filter_q1, int alpha, int beta, int lims, int lim_q1, int lim_p1)
Definition: rv34dsp.h:44
DSP utils.
rv34_inv_transform_func rv34_inv_transform
Definition: rv34dsp.h:68
h264_chroma_mc_func avg_chroma_pixels_tab[3]
Definition: rv34dsp.h:61
h264_chroma_mc_func put_chroma_pixels_tab[3]
Definition: rv34dsp.h:60
Generated on Mon Aug 4 2025 06:54:26 for FFmpeg by
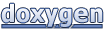