FFmpeg
|
postprocessing. More...
#include "config.h"
#include "libavutil/avutil.h"
#include "libavutil/avassert.h"
#include <inttypes.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "postprocess.h"
#include "postprocess_internal.h"
#include "libavutil/avstring.h"
#include "postprocess_template.c"
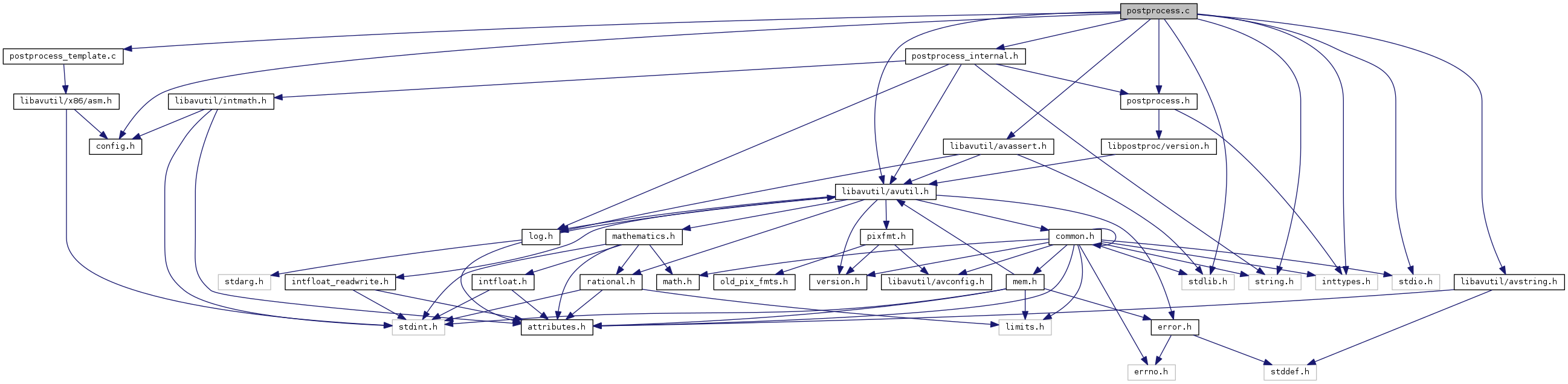
Go to the source code of this file.
Macros | |
#define | LICENSE_PREFIX "libpostproc license: " |
#define | GET_MODE_BUFFER_SIZE 500 |
#define | OPTIONS_ARRAY_SIZE 10 |
#define | BLOCK_SIZE 8 |
#define | TEMP_STRIDE 8 |
#define | TEMPLATE_PP_C 1 |
Typedefs | |
typedef void(* | pp_fn) (const uint8_t src[], int srcStride, uint8_t dst[], int dstStride, int width, int height, const QP_STORE_T QPs[], int QPStride, int isColor, PPContext *c2) |
Functions | |
unsigned | postproc_version (void) |
Return the LIBPOSTPROC_VERSION_INT constant. More... | |
const char * | postproc_configuration (void) |
Return the libpostproc build-time configuration. More... | |
const char * | postproc_license (void) |
Return the libpostproc license. More... | |
DECLARE_ASM_CONST (8, int, deringThreshold) | |
static int | isHorizDC_C (const uint8_t src[], int stride, const PPContext *c) |
Check if the given 8x8 Block is mostly "flat". More... | |
static int | isVertDC_C (const uint8_t src[], int stride, const PPContext *c) |
Check if the middle 8x8 Block in the given 8x16 block is flat. More... | |
static int | isHorizMinMaxOk_C (const uint8_t src[], int stride, int QP) |
static int | isVertMinMaxOk_C (const uint8_t src[], int stride, int QP) |
static int | horizClassify_C (const uint8_t src[], int stride, const PPContext *c) |
static int | vertClassify_C (const uint8_t src[], int stride, const PPContext *c) |
static void | doHorizDefFilter_C (uint8_t dst[], int stride, const PPContext *c) |
static void | doHorizLowPass_C (uint8_t dst[], int stride, const PPContext *c) |
Do a horizontal low pass filter on the 10x8 block (dst points to middle 8x8 Block) using the 9-Tap Filter (1,1,2,2,4,2,2,1,1)/16 (C version) More... | |
static void | horizX1Filter (uint8_t *src, int stride, int QP) |
Experimental Filter 1 (Horizontal) will not damage linear gradients Flat blocks should look like they were passed through the (1,1,2,2,4,2,2,1,1) 9-Tap filter can only smooth blocks at the expected locations (it cannot smooth them if they did move) MMX2 version does correct clipping C version does not not identical with the vertical one. More... | |
static av_always_inline void | do_a_deblock_C (uint8_t *src, int step, int stride, const PPContext *c) |
accurate deblock filter More... | |
static void | postProcess (const uint8_t src[], int srcStride, uint8_t dst[], int dstStride, int width, int height, const QP_STORE_T QPs[], int QPStride, int isColor, pp_mode *vm, pp_context *vc) |
pp_mode * | pp_get_mode_by_name_and_quality (const char *name, int quality) |
Return a pp_mode or NULL if an error occurred. More... | |
void | pp_free_mode (pp_mode *mode) |
static void | reallocAlign (void **p, int alignment, int size) |
static void | reallocBuffers (PPContext *c, int width, int height, int stride, int qpStride) |
static const char * | context_to_name (void *ptr) |
pp_context * | pp_get_context (int width, int height, int cpuCaps) |
void | pp_free_context (void *vc) |
void | pp_postprocess (const uint8_t *src[3], const int srcStride[3], uint8_t *dst[3], const int dstStride[3], int width, int height, const QP_STORE_T *QP_store, int QPStride, pp_mode *vm, void *vc, int pict_type) |
Variables | |
static const struct PPFilter | filters [] |
static const char * | replaceTable [] |
const char | pp_help [] |
a simple help text More... | |
static const AVClass | av_codec_context_class = { "Postproc", context_to_name, NULL } |
Detailed Description
postprocessing.
Definition in file postprocess.c.
Macro Definition Documentation
#define BLOCK_SIZE 8 |
Definition at line 115 of file postprocess.c.
Referenced by doHorizDefFilter_C(), doHorizLowPass_C(), horizX1Filter(), isHorizDC_C(), isVertDC_C(), and isVertMinMaxOk_C().
#define GET_MODE_BUFFER_SIZE 500 |
Definition at line 113 of file postprocess.c.
Referenced by pp_get_mode_by_name_and_quality().
#define LICENSE_PREFIX "libpostproc license: " |
Referenced by postproc_license().
#define OPTIONS_ARRAY_SIZE 10 |
Definition at line 114 of file postprocess.c.
Referenced by pp_get_mode_by_name_and_quality().
#define TEMP_STRIDE 8 |
Definition at line 116 of file postprocess.c.
#define TEMPLATE_PP_C 1 |
Definition at line 542 of file postprocess.c.
Typedef Documentation
typedef void(* pp_fn) (const uint8_t src[], int srcStride, uint8_t dst[], int dstStride, int width, int height, const QP_STORE_T QPs[], int QPStride, int isColor, PPContext *c2) |
Definition at line 578 of file postprocess.c.
Function Documentation
|
static |
Definition at line 889 of file postprocess.c.
DECLARE_ASM_CONST | ( | 8 | , |
int | , | ||
deringThreshold | |||
) |
|
static |
accurate deblock filter
Definition at line 438 of file postprocess.c.
Definition at line 302 of file postprocess.c.
Do a horizontal low pass filter on the 10x8 block (dst points to middle 8x8 Block) using the 9-Tap Filter (1,1,2,2,4,2,2,1,1)/16 (C version)
Definition at line 341 of file postprocess.c.
Definition at line 278 of file postprocess.c.
Experimental Filter 1 (Horizontal) will not damage linear gradients Flat blocks should look like they were passed through the (1,1,2,2,4,2,2,1,1) 9-Tap filter can only smooth blocks at the expected locations (it cannot smooth them if they did move) MMX2 version does correct clipping C version does not not identical with the vertical one.
Definition at line 381 of file postprocess.c.
Referenced by postProcess().
Check if the given 8x8 Block is mostly "flat".
Definition at line 204 of file postprocess.c.
Referenced by horizClassify_C().
|
inlinestatic |
Definition at line 249 of file postprocess.c.
Referenced by horizClassify_C().
Check if the middle 8x8 Block in the given 8x16 block is flat.
Definition at line 227 of file postprocess.c.
Referenced by vertClassify_C().
|
inlinestatic |
Definition at line 265 of file postprocess.c.
Referenced by vertClassify_C().
|
inlinestatic |
Definition at line 581 of file postprocess.c.
Referenced by pp_postprocess().
Definition at line 857 of file postprocess.c.
Referenced by reallocBuffers().
|
static |
Definition at line 862 of file postprocess.c.
Referenced by pp_get_context(), and pp_postprocess().
Definition at line 290 of file postprocess.c.
Variable Documentation
Definition at line 893 of file postprocess.c.
Referenced by pp_get_context().
|
static |
Definition at line 133 of file postprocess.c.
|
static |
Definition at line 157 of file postprocess.c.
Referenced by pp_get_mode_by_name_and_quality().
Generated on Sun Mar 9 2025 06:53:40 for FFmpeg by
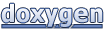