FFmpeg
|
libavformat/h261dec.c
Go to the documentation of this file.
#define FF_DEF_RAWVIDEO_DEMUXER(shortname, longname, probe, ext, id)
Definition: rawdec.h:52
bitstream reader API header.
Definition: libavcodec/avcodec.h:106
unsigned char * buf
Buffer must have AVPROBE_PADDING_SIZE of extra allocated bytes filled with zero.
Definition: avformat.h:336
or the Software in violation of any applicable export control laws in any jurisdiction Except as provided by mandatorily applicable UPF has no obligation to provide you with source code to the Software In the event Software contains any source code
Definition: MELODIA - License.txt:24
static int init_get_bits(GetBitContext *s, const uint8_t *buffer, int bit_size)
Initialize GetBitContext.
Definition: get_bits.h:379
This structure contains the data a format has to probe a file.
Definition: avformat.h:334
Main libavformat public API header.
Definition: get_bits.h:54
Generated on Fri Jun 20 2025 06:52:31 for FFmpeg by
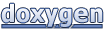