FFmpeg
|
IFF ACBM/DEEP/ILBM/PBM bitmap decoder. More...
#include "libavutil/imgutils.h"
#include "bytestream.h"
#include "avcodec.h"
#include "get_bits.h"
#include "internal.h"
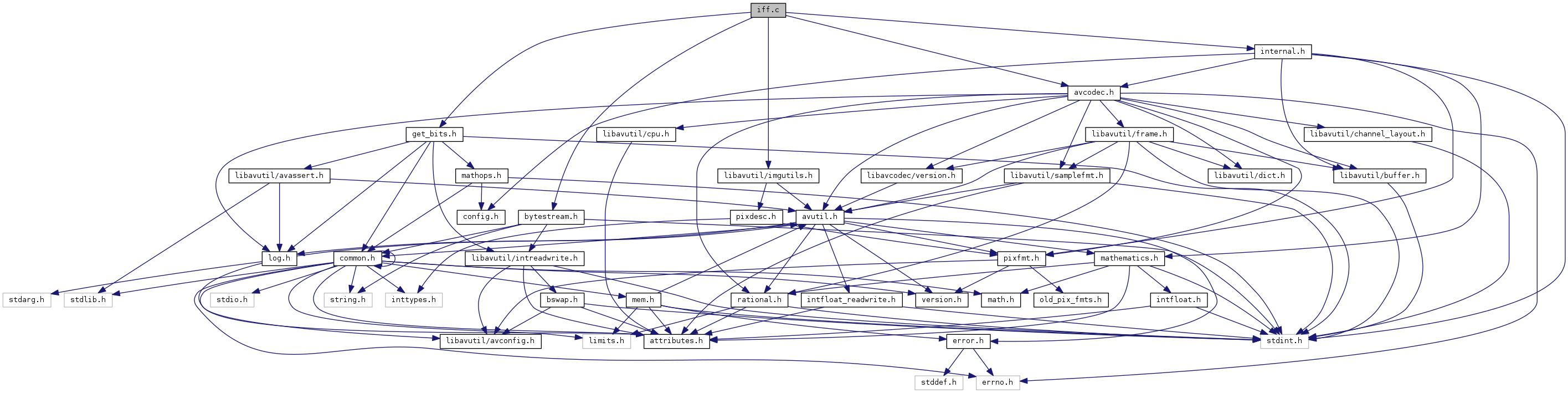
Go to the source code of this file.
Data Structures | |
struct | IffContext |
Macros | |
#define | LUT8_PART(plane, v) |
#define | LUT8(plane) |
#define | LUT32(plane) |
#define | DECODE_HAM_PLANE32(x) |
#define | DECODE_RGBX_COMMON(type) |
#define | GETNIBBLE ((i & 1) ? (src[i>>1] & 0xF) : (src[i>>1] >> 4)) |
Enumerations | |
enum | mask_type { MASK_NONE, MASK_HAS_MASK, MASK_HAS_TRANSPARENT_COLOR, MASK_LASSO } |
Functions | |
static av_always_inline uint32_t | gray2rgb (const uint32_t x) |
static int | cmap_read_palette (AVCodecContext *avctx, uint32_t *pal) |
Convert CMAP buffer (stored in extradata) to lavc palette format. More... | |
static int | extract_header (AVCodecContext *const avctx, const AVPacket *const avpkt) |
Extracts the IFF extra context and updates internal decoder structures. More... | |
static av_cold int | decode_init (AVCodecContext *avctx) |
static void | decodeplane8 (uint8_t *dst, const uint8_t *buf, int buf_size, int plane) |
Decode interleaved plane buffer up to 8bpp. More... | |
static void | decodeplane32 (uint32_t *dst, const uint8_t *buf, int buf_size, int plane) |
Decode interleaved plane buffer up to 24bpp. More... | |
static void | decode_ham_plane32 (uint32_t *dst, const uint8_t *buf, const uint32_t *const pal, unsigned buf_size) |
Converts one line of HAM6/8-encoded chunky buffer to 24bpp. More... | |
static void | lookup_pal_indicies (uint32_t *dst, const uint32_t *buf, const uint32_t *const pal, unsigned width) |
static int | decode_byterun (uint8_t *dst, int dst_size, const uint8_t *buf, const uint8_t *const buf_end) |
Decode one complete byterun1 encoded line. More... | |
static void | decode_rgb8 (GetByteContext *gb, uint8_t *dst, int width, int height, int linesize) |
Decode RGB8 buffer. More... | |
static void | decode_rgbn (GetByteContext *gb, uint8_t *dst, int width, int height, int linesize) |
Decode RGBN buffer. More... | |
static void | decode_deep_rle32 (uint8_t *dst, const uint8_t *src, int src_size, int width, int height, int linesize) |
Decode DEEP RLE 32-bit buffer. More... | |
static void | decode_deep_tvdc32 (uint8_t *dst, const uint8_t *src, int src_size, int width, int height, int linesize, const int16_t *tvdc) |
Decode DEEP TVDC 32-bit buffer. More... | |
static int | unsupported (AVCodecContext *avctx) |
static int | decode_frame (AVCodecContext *avctx, void *data, int *got_frame, AVPacket *avpkt) |
static av_cold int | decode_end (AVCodecContext *avctx) |
Variables | |
static const uint64_t | plane8_lut [8][256] |
static const uint32_t | plane32_lut [32][16 *4] |
Detailed Description
IFF ACBM/DEEP/ILBM/PBM bitmap decoder.
Definition in file libavcodec/iff.c.
Macro Definition Documentation
#define DECODE_HAM_PLANE32 | ( | x | ) |
Definition at line 420 of file libavcodec/iff.c.
Referenced by decode_ham_plane32().
#define DECODE_RGBX_COMMON | ( | type | ) |
Definition at line 492 of file libavcodec/iff.c.
Referenced by decode_rgb8(), and decode_rgbn().
Referenced by decode_deep_tvdc32().
#define LUT32 | ( | plane | ) |
Definition at line 103 of file libavcodec/iff.c.
#define LUT8 | ( | plane | ) |
Definition at line 78 of file libavcodec/iff.c.
#define LUT8_PART | ( | plane, | |
v | |||
) |
Definition at line 60 of file libavcodec/iff.c.
Enumeration Type Documentation
enum mask_type |
Enumerator | |
---|---|
MASK_NONE | |
MASK_HAS_MASK | |
MASK_HAS_TRANSPARENT_COLOR | |
MASK_LASSO |
Definition at line 35 of file libavcodec/iff.c.
Function Documentation
|
static |
Convert CMAP buffer (stored in extradata) to lavc palette format.
Definition at line 142 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Decode one complete byterun1 encoded line.
- Parameters
-
dst the destination buffer where to store decompressed bitstream dst_size the destination plane size in bytes buf the source byterun1 compressed bitstream buf_end the EOF of source byterun1 compressed bitstream
- Returns
- number of consumed bytes in byterun1 compressed bitstream
Definition at line 470 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Decode DEEP RLE 32-bit buffer.
- Parameters
-
[out] dst Destination buffer [in] src Source buffer src_size Source buffer size (bytes) width Width of destination buffer (pixels) height Height of destination buffer (pixels) linesize Line size of destination buffer (bytes)
Definition at line 556 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Decode DEEP TVDC 32-bit buffer.
- Parameters
-
[out] dst Destination buffer [in] src Source buffer src_size Source buffer size (bytes) width Width of destination buffer (pixels) height Height of destination buffer (pixels) linesize Line size of destination buffer (bytes)
Definition at line 606 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Definition at line 861 of file libavcodec/iff.c.
|
static |
Definition at line 653 of file libavcodec/iff.c.
Referenced by decode_end().
|
static |
Converts one line of HAM6/8-encoded chunky buffer to 24bpp.
- Parameters
-
dst the destination 24bpp buffer buf the source 8bpp chunky buffer pal the HAM decode table buf_size the plane size in bytes
Definition at line 438 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Definition at line 323 of file libavcodec/iff.c.
Referenced by decode_end().
|
static |
Decode RGB8 buffer.
- Parameters
-
[out] dst Destination buffer width Width of destination buffer (pixels) height Height of destination buffer (pixels) linesize Line size of destination buffer (bytes)
Definition at line 519 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Decode RGBN buffer.
- Parameters
-
[out] dst Destination buffer width Width of destination buffer (pixels) height Height of destination buffer (pixels) linesize Line size of destination buffer (bytes)
Definition at line 536 of file libavcodec/iff.c.
Referenced by decode_frame().
Decode interleaved plane buffer up to 24bpp.
- Parameters
-
dst Destination buffer buf Source buffer buf_size plane plane number to decode as
Definition at line 402 of file libavcodec/iff.c.
Referenced by decode_frame().
Decode interleaved plane buffer up to 8bpp.
- Parameters
-
dst Destination buffer buf Source buffer buf_size plane plane number to decode as
Definition at line 381 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Extracts the IFF extra context and updates internal decoder structures.
- Parameters
-
avctx the AVCodecContext where to extract extra context to avpkt the AVPacket to extract extra context from or NULL to use avctx
- Returns
- 0 in case of success, a negative error code otherwise
Definition at line 191 of file libavcodec/iff.c.
Referenced by decode_frame(), and decode_init().
|
static |
Definition at line 135 of file libavcodec/iff.c.
Referenced by cmap_read_palette(), and extract_header().
|
static |
Definition at line 453 of file libavcodec/iff.c.
Referenced by decode_frame().
|
static |
Definition at line 646 of file libavcodec/iff.c.
Referenced by decode_frame(), mov_read_elst(), and txd_decode_frame().
Variable Documentation
|
static |
|
static |
Generated on Wed Apr 16 2025 06:53:27 for FFmpeg by
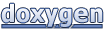