FFmpeg
|
dv1394.c File Reference
#include "config.h"
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
#include <poll.h>
#include <sys/ioctl.h>
#include <sys/mman.h>
#include "libavutil/log.h"
#include "libavutil/opt.h"
#include "avdevice.h"
#include "libavformat/dv.h"
#include "dv1394.h"
Include dependency graph for dv1394.c:
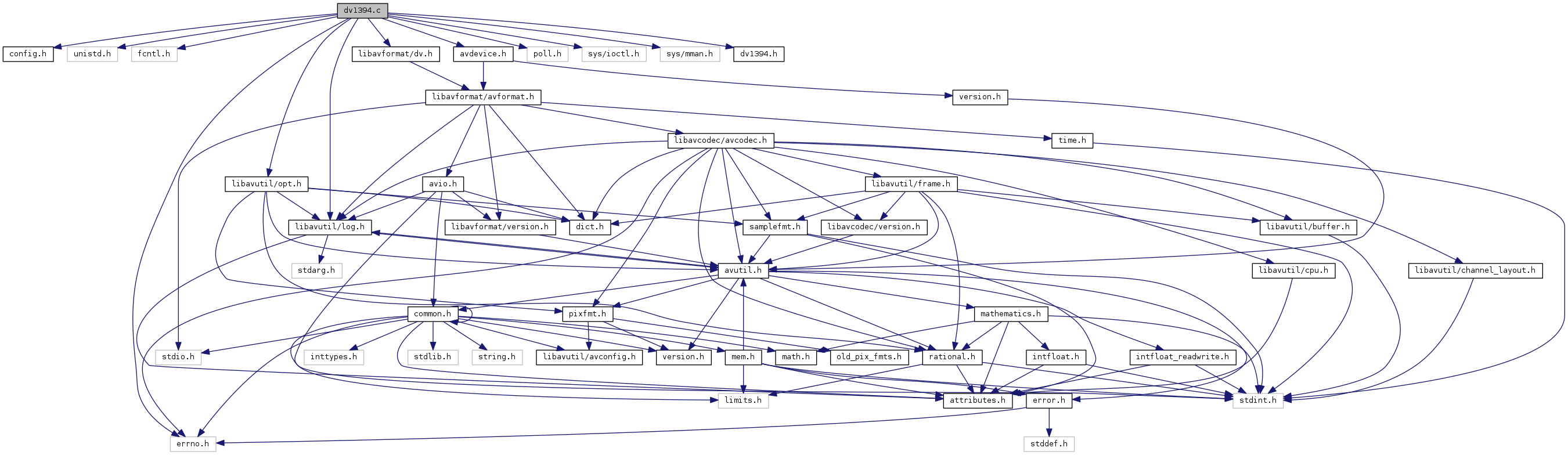
Go to the source code of this file.
Data Structures | |
struct | dv1394_data |
Functions | |
static int | dv1394_reset (struct dv1394_data *dv) |
static int | dv1394_start (struct dv1394_data *dv) |
static int | dv1394_read_header (AVFormatContext *context) |
static int | dv1394_read_packet (AVFormatContext *context, AVPacket *pkt) |
static int | dv1394_close (AVFormatContext *context) |
Variables | |
static const AVOption | options [] |
static const AVClass | dv1394_class |
AVInputFormat | ff_dv1394_demuxer |
Function Documentation
|
static |
|
static |
|
static |
|
static |
Definition at line 56 of file dv1394.c.
Referenced by dv1394_read_header(), and dv1394_read_packet().
|
static |
Definition at line 72 of file dv1394.c.
Referenced by dv1394_read_header(), and dv1394_read_packet().
Variable Documentation
|
static |
Initial value:
= {
.class_name = "DV1394 indev",
.item_name = av_default_item_name,
.option = options,
.version = LIBAVUTIL_VERSION_INT,
}
AVInputFormat ff_dv1394_demuxer |
Initial value:
= {
.name = "dv1394",
.long_name = NULL_IF_CONFIG_SMALL("DV1394 A/V grab"),
.read_packet = dv1394_read_packet,
.read_close = dv1394_close,
.flags = AVFMT_NOFILE,
.priv_class = &dv1394_class,
}
Definition: dv1394.c:36
static int dv1394_read_packet(AVFormatContext *context, AVPacket *pkt)
Definition: dv1394.c:119
#define NULL_IF_CONFIG_SMALL(x)
Return NULL if CONFIG_SMALL is true, otherwise the argument without modification. ...
Definition: libavutil/internal.h:123
#define AVFMT_NOFILE
Demuxer will use avio_open, no opened file should be provided by the caller.
Definition: avformat.h:345
|
static |
Initial value:
= {
{ "standard", "", offsetof(struct dv1394_data, format), AV_OPT_TYPE_INT, {.i64 = DV1394_NTSC}, DV1394_NTSC, DV1394_PAL, AV_OPT_FLAG_DECODING_PARAM, "standard" },
{ "PAL", "", 0, AV_OPT_TYPE_CONST, {.i64 = DV1394_PAL}, 0, 0, AV_OPT_FLAG_DECODING_PARAM, "standard" },
{ "NTSC", "", 0, AV_OPT_TYPE_CONST, {.i64 = DV1394_NTSC}, 0, 0, AV_OPT_FLAG_DECODING_PARAM, "standard" },
{ "channel", "", offsetof(struct dv1394_data, channel), AV_OPT_TYPE_INT, {.i64 = DV1394_DEFAULT_CHANNEL}, 0, INT_MAX, AV_OPT_FLAG_DECODING_PARAM },
{ NULL },
}
Definition: dv1394.c:36
Definition: opt.h:222
Definition: dv1394.h:260
Definition: opt.h:229
Filter the word “frame” indicates either a video frame or a group of audio as stored in an AVFilterBuffer structure Format for each input and each output the list of supported formats For video that means pixel format For audio that means channel sample format(the sample packing is implied by the sample format) and sample rate.The lists are not just lists
#define AV_OPT_FLAG_DECODING_PARAM
a generic parameter which can be set by the user for demuxing or decoding
Definition: opt.h:282
Definition: dv1394.h:259
Generated on Sat Feb 22 2025 06:54:46 for FFmpeg by
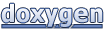