x
|
Go to the source code of this file.
Functions | |
void | Daubechies (int p, double *h) |
void | splinewl (int p1, int p2, double *h1, double *h2) |
int | splinewl (int p1, int p2, double *h, double *hm, double *g, double *gm, int normmode=0, int *points=0) |
int | dwtpqmf (double *in, int Count, int N, double *h, double *g, int M, double *out) |
int | dwtp (double *in, int Count, int N, double *h, double *g, int M, double *out) |
int | dwtp (double *in, int Count, int N, double *h, int Mh, double *g, int Mg, double *out) |
int | dwtp (double *in, int Count, int N, double *h, int sh, int eh, double *g, int sg, int eg, double *out) |
void | idwtp (double *in, int Count, int N, double *h, double *g, int M, double *out) |
void | idwtp (double *in, int Count, int N, double *h, int Mh, double *g, int Mg, double *out) |
void | idwtp (double *in, int Count, int N, double *h, int sh, int eh, double *g, int sg, int eg, double *out) |
void | wavpacqmf (double ***spec, double *data, int Count, int WID, int wid, int M, double *h, double *g) |
void | wavpac (double ***spec, double *data, int Count, int WID, int wid, double *h, int hs, int he, double *g, int gs, int ge) |
void | iwavpacqmf (double *data, double **spec, int Fr, int Wid, int M, double *h, double *g) |
void | iwavpac (double *data, double **spec, int Fr, int Wid, double *h, int hs, int he, double *g, int gs, int ge) |
Detailed Description
- wavelet routines
Function Documentation
void Daubechies | ( | int | p, |
double * | h | ||
) |
function Daubechies: calculates the Daubechies filter of a given order p
In: filter order p Out: h[2p]: the 2p FIR coefficients
No reutrn value. The calculated filters are minimum phase, which means the energy concentrates at the beginning. This is usually used for reconstruction. On the contrary, for wavelet analysis the filter is mirrored.
int dwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
double * | g, | ||
int | M, | ||
double * | out | ||
) |
function dwtp: in this implementation h and g can be different from mirrored reconstruction filters, i.e. the biorthogonal transform. h[0] and g[0] are aligned at the ends of the filters, i.e. h[-M+1:0], g[-M+1:0].
In: in[Count]: waveform data h[-M+1:0], g[-M+1:0]: quadratic mirror filter pair N: maximal time resolution Out: out[N], the wavelet transform, arranged in interleaved format.
Returns maximal atom length (should equal N).
int dwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
int | Mh, | ||
double * | g, | ||
int | Mg, | ||
double * | out | ||
) |
function dwtp: in this implementation h and g can be different size. h[0] and g[0] are aligned at the ends of the filters, i.e. h[-Mh+1:0], g[-Mg+1:0].
In: in[Count]: waveform data h[-Mh+1:0], g[-Mg+1:0]: quadratic mirror filter pair N: maximal time resolution Out: out[N], the wavelet transform, arranged in interleaved format.
Returns maximal atom length (should equal N).
int dwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
int | sh, | ||
int | eh, | ||
double * | g, | ||
int | sg, | ||
int | eg, | ||
double * | out | ||
) |
function dwtp: in this implementation h and g can be arbitrarily located: h from $sh to $eh, g from $sg to $eg.
In: in[Count]: waveform data h[sh:eh-1], g[sg:eg-1]: quadratic mirror filter pair N: maximal time resolution Out: out[N], the wavelet transform, arranged in interleaved format.
Returns maximal atom length (should equal N).
int dwtpqmf | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
double * | g, | ||
int | M, | ||
double * | out | ||
) |
function dwtpqmf: in this implementation h and g are the same as reconstruction qmf filters. In fact the actual filters used are their mirrors and filter origin are aligned to the ends of the real filters, which turn out to be the starts of h and g.
The inverse transform is idwtp(), the same as inversing dwtp().
In: in[Count]: waveform data h[M], g[M]: quadratic mirror filter pair N: maximal time resolution Count=kN, N=2^lN being the reciprocal of the most detailed frequency scale, i.e. N=1 for no transforming at all, N=2 for dividing into approx. and detail, N=4 for dividing into approx+detail(approx+detial), etc. Count*2/N=2k gives the smallest length to be convolved with h and g. Out: out[N], the wavelet transform, arranged in interleaved format.
Returns maximal atom length (should equal N).
void idwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
double * | g, | ||
int | M, | ||
double * | out | ||
) |
function idwtp: periodic wavelet reconstruction by qmf
In: in[Count]: wavelet transform in interleaved format h[M], g[M]: quadratic mirror filter pair N: maximal time resolution Out: out[N], waveform data (detail level 0).
No return value.
void idwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
int | Mh, | ||
double * | g, | ||
int | Mg, | ||
double * | out | ||
) |
function idwtp: in which h and g can have different length.
In: in[Count]: wavelet transform in interleaved format h[Mh], g[Mg]: quadratic mirror filter pair N: maximal time resolution Out: out[N], waveform data (detail level 0).
No return value.
void idwtp | ( | double * | in, |
int | Count, | ||
int | N, | ||
double * | h, | ||
int | sh, | ||
int | eh, | ||
double * | g, | ||
int | sg, | ||
int | eg, | ||
double * | out | ||
) |
function idwtp: in which h and g can be arbitrarily located: h from $sh to $eh, g from $sg to $eg
In: in[Count]: wavelet transform in interleaved format h[sh:eh-1], g[sg:eg-1]: quadratic mirror filter pair N: maximal time resolution Out: out[N], waveform data (detail level 0).
No return value.
void iwavpac | ( | double * | data, |
double ** | spec, | ||
int | Fr, | ||
int | Wid, | ||
double * | h, | ||
int | hs, | ||
int | he, | ||
double * | g, | ||
int | gs, | ||
int | ge | ||
) |
function iwavpac: inverse transform of wavpac
In: spec[Fr][Wid] h[hs:he-1], g[gs:ge-1], reconstruction filter pair Out: data[Fr*Wid]
No return value.
void iwavpacqmf | ( | double * | data, |
double ** | spec, | ||
int | Fr, | ||
int | Wid, | ||
int | M, | ||
double * | h, | ||
double * | g | ||
) |
function iwavpacqmf: inverse transform of wavpacqmf
In: spec[Fr][Wid], Fr>M*2 h[M], g[M], quadratic mirror filter pair Out: data[Fr*Wid]
No return value.
void splinewl | ( | int | p1, |
int | p2, | ||
double * | h, | ||
double * | hm | ||
) |
function splinewl: computes spline biorthogonal wavelet filters. This version of splinewl calcualtes the positive-time half of h and hm coefficients only.
p1 and p2 must have the same parity. If p1 is even, p1 coefficients will be returned in h1; if p1 is odd, p1-1 coefficients will be returned in h1.
Actual length of h is p1+1, of hm is p1+2p2-1. only a half of each is kept. p even: h[0:p1/2] <- [p1/2:p1], hm[0:p1/2+p2-1] <- [p1/2+p2-1:p1+2p2-2] p odd: h[0:(p1-1)/2] <- [(p1+1)/2:p1], hm[0:(p1-3)/2+p2] <- [(p1-1)/2+p2:p1+2p2-2] i.e. h[0:hp1] <- [p1-hp1:p1], hm[0:hp1+p2-1] <- [p1-hp1-1+p2:p1+2p2-2]
In: p1, p2: specify vanishing moments of h and hm Out: h[] and hm[] as specified above.
No return value.
int splinewl | ( | int | p1, |
int | p2, | ||
double * | h, | ||
double * | hm, | ||
double * | g, | ||
double * | gm, | ||
int | normmode, | ||
int * | points | ||
) |
function splinewl: calculates the analysis and reconstruction filter pairs of spline biorthogonal wavelet (h, g) and (hm, gm). h has the size p1+1, hm has the size p1+2p2-1.
If p1+1 is odd, then all four filters are symmetric; if not, then h and hm are symmetric, while g and gm are anti-symmetric.
The concatenation of filters h with hm (or g with gm) introduces a time shift of p1+p2-1, which is the return value multiplied by -1.
If normmode==1, the results are normalized so that ||h||^2=||g||^2=1; if normmode==2, the results are normalized so that ||hm||^2=||gm||^2=1, if normmode==3, the results are normalized so that ||h||^2==||g||^2=||hm||^2=||gm||^2.
If a points buffer is specified, the function returns the starting and ending positions (inclusive) of h, hm, g, and gm, in the order of (hs, he, hms, hme, gs, ge, gms, gme), as ps[0]~ps[7].
In: p1 and p2, specify vanishing moments of h and hm, respectively. normmode: mode for normalization Out: h[p1+1], g[p1+1], hm[p1+2p2-1], gm[p1+2p2-1], points[8] (optional)
Returns -p1-p2+1.
void wavpac | ( | double *** | spec, |
double * | data, | ||
int | Count, | ||
int | WID, | ||
int | wid, | ||
double * | h, | ||
int | hs, | ||
int | he, | ||
double * | g, | ||
int | gs, | ||
int | ge | ||
) |
function wavpac: calculate pseudo local cosine transforms using wavelet packet,
In: data[Count], Count=fr*WID, waveform data WID: largest scale, equals 2^ORDER wid: smallest scale, euqals 2^order h[hs:he-1], g[gs:ge-1]: filter pair Out: spec[0][fr][WID], spec[1][2*fr][WID/2], ..., spec[ORDER-order-1][FR][wid]
No return value.
void wavpacqmf | ( | double *** | spec, |
double * | data, | ||
int | Count, | ||
int | WID, | ||
int | wid, | ||
int | M, | ||
double * | h, | ||
double * | g | ||
) |
function wavpacqmf: calculate pseudo local cosine transforms using wavelet packet
In: data[Count], Count=fr*WID, waveform data WID: largest scale, equals 2^ORDER wid: smallest scale, euqals 2^order h[M], g[M]: quadratic mirror filter pair, fr>2*M Out: spec[0][fr][WID], spec[1][2*fr][WID/2], ..., spec[ORDER-order-1][FR][wid]
No return value.
Generated on Thu Feb 20 2025 07:06:32 for x by
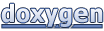