x
|
wavelet.h
Go to the documentation of this file.
22 void splinewl(int p1, int p2, double* h1, double* h2); //compute spline biorthogonal wavelet filter
23 int splinewl(int p1, int p2, double* h, double* hm, double* g, double* gm, int normmode=0, int* points=0);//compute spline biorthogonal wavelet filter
29 int dwtp(double* in, int Count, int N, double* h, int sh, int eh, double* g, int sg, int eg, double* out);
32 void idwtp(double* in, int Count, int N, double* h, int sh, int eh, double* g, int sg, int eg, double* out);
35 void wavpacqmf(double*** spec, double* data, int Count, int WID, int wid, int M, double* h, double* g);
36 void wavpac(double*** spec, double* data, int Count, int WID, int wid, double* h, int hs, int he, double* g, int gs, int ge);
38 void iwavpac(double* data, double** spec, int Fr, int Wid, double* h, int hs, int he, double* g, int gs, int ge);
int dwtpqmf(double *in, int Count, int N, double *h, double *g, int M, double *out)
Definition: wavelet.cpp:138
void wavpac(double ***spec, double *data, int Count, int WID, int wid, double *h, int hs, int he, double *g, int gs, int ge)
Definition: wavelet.cpp:881
void iwavpac(double *data, double **spec, int Fr, int Wid, double *h, int hs, int he, double *g, int gs, int ge)
Definition: wavelet.cpp:965
void wavpacqmf(double ***spec, double *data, int Count, int WID, int wid, int M, double *h, double *g)
Definition: wavelet.cpp:757
void idwtp(double *in, int Count, int N, double *h, double *g, int M, double *out)
Definition: wavelet.cpp:372
void iwavpacqmf(double *data, double **spec, int Fr, int Wid, int M, double *h, double *g)
Definition: wavelet.cpp:822
int dwtp(double *in, int Count, int N, double *h, double *g, int M, double *out)
Definition: wavelet.cpp:198
Generated on Tue Apr 1 2025 07:12:14 for x by
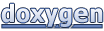