VampPluginSDK
2.10
|
plugins.cpp
Go to the documentation of this file.
PluginAdapter turns a PluginAdapterBase into a specific wrapper for a particular plugin implementatio...
Definition: PluginAdapter.h:95
static Vamp::PluginAdapter< PercussionOnsetDetector > percussionOnsetAdapter
Definition: plugins.cpp:49
const VampPluginDescriptor * getDescriptor()
Return a VampPluginDescriptor describing the plugin that is wrapped by this adapter.
static Vamp::PluginAdapter< ZeroCrossing > zeroCrossingAdapter
Definition: plugins.cpp:47
static Vamp::PluginAdapter< SpectralCentroid > spectralCentroidAdapter
Definition: plugins.cpp:48
Definition: vamp.h:248
static Vamp::PluginAdapter< AmplitudeFollower > amplitudeAdapter
Definition: plugins.cpp:51
const VampPluginDescriptor * vampGetPluginDescriptor(unsigned int version, unsigned int index)
Get the descriptor for a given plugin index in this library.
Definition: plugins.cpp:54
static Vamp::PluginAdapter< FixedTempoEstimator > fixedTempoAdapter
Definition: plugins.cpp:50
Generated by
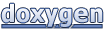