svgui
1.9
|
WindowTypeSelector.cpp
Go to the documentation of this file.
Definition: WindowShapePreview.h:25
void windowTypeChanged(WindowType type)
WindowShapePreview * m_windowShape
Definition: WindowTypeSelector.h:48
void windowIndexChanged(int index)
Definition: WindowTypeSelector.cpp:111
virtual ~WindowTypeSelector()
Definition: WindowTypeSelector.cpp:86
void setWindowType(WindowType type)
Definition: WindowTypeSelector.cpp:98
void setWindowType(WindowType type)
Definition: WindowShapePreview.cpp:215
WindowType getWindowType() const
Definition: WindowTypeSelector.cpp:92
Generated by
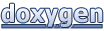