svgui
1.9
|
UnitConverter.h
Go to the documentation of this file.
Definition: UnitConverter.h:27
void preferenceChanged(PropertyContainer::PropertyName)
Definition: UnitConverter.cpp:265
Generated by
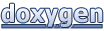