svgui
1.9
|
TransformFinder.h
Go to the documentation of this file.
Definition: SelectableLabel.h:21
std::vector< TextMatcher::Match > SortedResults
Definition: TransformFinder.h:66
void searchTextChanged(const QString &)
Definition: TransformFinder.cpp:170
void setupBeforeSearchLabel()
Definition: TransformFinder.cpp:123
TransformFinder(QWidget *parent=0)
Definition: TransformFinder.cpp:33
Definition: TransformFinder.h:33
Generated by
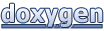