svgui
1.9
|
TipDialog.h
Go to the documentation of this file.
bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName) override
Definition: TipDialog.cpp:232
TipFileParser(TipDialog *dialog)
Definition: TipDialog.cpp:163
bool error(const QXmlParseException &exception) override
Definition: TipDialog.cpp:276
bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts) override
Definition: TipDialog.cpp:185
bool fatalError(const QXmlParseException &exception) override
Definition: TipDialog.cpp:288
Definition: TipDialog.h:30
Definition: TipDialog.h:54
bool characters(const QString &) override
Definition: TipDialog.cpp:264
Generated by
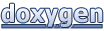