svgui
1.9
|
Thumbwheel.h
Go to the documentation of this file.
void contextMenuRequested(const QPoint &)
Definition: Thumbwheel.cpp:68
Thumbwheel(Qt::Orientation orientation, QWidget *parent=0)
Definition: Thumbwheel.cpp:34
void mouseDoubleClickEvent(QMouseEvent *e) override
Definition: Thumbwheel.cpp:373
Definition: Thumbwheel.h:29
Manage the little bit of tedious book-keeping associated with translating vertical wheel events into ...
Definition: WheelCounter.h:24
void mouseReleaseEvent(QMouseEvent *e) override
Definition: Thumbwheel.cpp:477
void mousePressEvent(QMouseEvent *e) override
Definition: Thumbwheel.cpp:359
void mouseMoveEvent(QMouseEvent *e) override
Definition: Thumbwheel.cpp:452
void setProvideContextMenu(bool provide)
Definition: Thumbwheel.cpp:116
void mouseLeft()
void valueChanged(int)
void mouseEntered()
Generated by
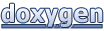