svgui
1.9
|
SubdividingMenu.h
Go to the documentation of this file.
std::map< QString, QObject * > m_pendingEntries
Definition: SubdividingMenu.h:72
void setEntries(const std::set< QString > &entries)
Definition: SubdividingMenu.cpp:61
A menu that divides its entries into submenus, alphabetically.
Definition: SubdividingMenu.h:37
SubdividingMenu(int lowerLimit=0, int upperLimit=0, QWidget *parent=0)
Definition: SubdividingMenu.cpp:27
std::map< QString, QMenu * > m_nameToChunkMenuMap
Definition: SubdividingMenu.h:66
Generated by
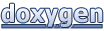