svgui
1.9
|
ProgressDialog.cpp
Go to the documentation of this file.
void setDefinite(bool definite) override
Definition: ProgressDialog.cpp:65
void cancelled()
void setProgress(int percentage) override
Definition: ProgressDialog.cpp:102
ProgressDialog(QString message, bool cancellable, int timeBeforeShow=0, QWidget *parent=0, Qt::WindowModality modality=Qt::NonModal)
Definition: ProgressDialog.cpp:22
void showing()
Generated by
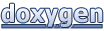