svgui
1.9
|
ModelDataTableDialog.h
Go to the documentation of this file.
Definition: ModelDataTableDialog.h:32
void currentChangedThroughResort(const QModelIndex &)
Definition: ModelDataTableDialog.cpp:340
void playbackScrolledToFrame(sv_frame_t frame)
Definition: ModelDataTableDialog.cpp:192
void scrollToFrame(sv_frame_t frame)
ModelDataTableDialog(ModelId tabularModelId, QString title, QWidget *parent=0)
Definition: ModelDataTableDialog.cpp:41
void togglePlayTracking()
Definition: ModelDataTableDialog.cpp:334
QToolBar * getPlayToolbar()
Definition: ModelDataTableDialog.h:41
~ModelDataTableDialog()
Definition: ModelDataTableDialog.cpp:159
void viewPressed(const QModelIndex &)
Definition: ModelDataTableDialog.cpp:274
void viewClicked(const QModelIndex &)
Definition: ModelDataTableDialog.cpp:264
void userScrolledToFrame(sv_frame_t frame)
Definition: ModelDataTableDialog.cpp:165
void currentChanged(const QModelIndex &, const QModelIndex &)
Definition: ModelDataTableDialog.cpp:282
void searchTextChanged(const QString &)
Definition: ModelDataTableDialog.cpp:201
Generated by
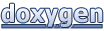