svgui
1.9
|
MIDIFileImportDialog.cpp
Go to the documentation of this file.
51 tr("<b>Select track to import</b><p>You can only import this file as a single annotation layer, but the file contains more than one track, or notes on more than one channel.<p>Please select the track or merged tracks you wish to import:"),
void showError(QString error) override
Definition: MIDIFileImportDialog.cpp:68
MIDIFileImportDialog(QWidget *parent=0)
Definition: MIDIFileImportDialog.cpp:20
TrackPreference getTrackImportPreference(QStringList trackNames, bool haveSomePercussion, QString &singleTrack) const override
Definition: MIDIFileImportDialog.cpp:26
Generated by
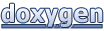