svgui
1.9
|
ListInputDialog.h
Go to the documentation of this file.
std::vector< QRadioButton * > m_radioButtons
Definition: ListInputDialog.h:54
ListInputDialog(QWidget *parent, const QString &title, const QString &label, const QStringList &list, int current=0)
Definition: ListInputDialog.cpp:26
Like QInputDialog::getItem(), except that it offers the items as a set of radio buttons instead of in...
Definition: ListInputDialog.h:33
QString getCurrentString() const
Definition: ListInputDialog.cpp:66
void setItemAvailability(int item, bool available)
Definition: ListInputDialog.cpp:77
static QString getItem(QWidget *parent, const QString &title, const QString &label, const QStringList &list, int current=0, bool *ok=0)
Definition: ListInputDialog.cpp:90
Generated by
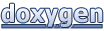