svgui
1.9
|
LabelCounterInputDialog.cpp
Go to the documentation of this file.
int m_origSecondCounter
Definition: LabelCounterInputDialog.h:38
void cancelClicked()
Definition: LabelCounterInputDialog.cpp:83
virtual ~LabelCounterInputDialog()
Definition: LabelCounterInputDialog.cpp:66
void secondCounterChanged(int)
Definition: LabelCounterInputDialog.cpp:77
void counterChanged(int)
Definition: LabelCounterInputDialog.cpp:71
LabelCounterInputDialog(Labeller *labeller, QWidget *parent)
Definition: LabelCounterInputDialog.cpp:24
Generated by
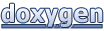