svgui
1.9
|
KeyReference.h
Go to the documentation of this file.
Definition: KeyReference.h:55
std::vector< QString > alternatives
Definition: KeyReference.h:59
Definition: KeyReference.h:30
void dialogButtonClicked(QAbstractButton *)
Definition: KeyReference.cpp:206
void registerAlternativeShortcut(QAction *, QString alternative)
Definition: KeyReference.cpp:84
void registerShortcut(QAction *, QString overrideName="")
Definition: KeyReference.cpp:48
Generated by
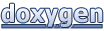