svgui
1.9
|
ImageDialog.h
Go to the documentation of this file.
Definition: ImageDialog.h:27
void labelChanged(QString label)
void imageChanged(QString image)
ImageDialog(QString title, QString image="", QString label="", QWidget *parent=0)
Definition: ImageDialog.cpp:36
Generated by
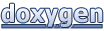