svgui
1.9
|
CSVFormatDialog.h
Go to the documentation of this file.
void windowSizeChanged(QString)
Definition: CSVFormatDialog.cpp:473
void updateComboVisibility()
Definition: CSVFormatDialog.cpp:408
void columnPurposeChangedForAnnotationType(QComboBox *, int purpose)
void timingTypeChanged(int type)
Definition: CSVFormatDialog.cpp:452
TimingOption m_initialTimingOption
Definition: CSVFormatDialog.h:71
sv_samplerate_t m_referenceSampleRate
Definition: CSVFormatDialog.h:60
Definition: CSVFormatDialog.h:29
void applyStartTimePurpose()
Definition: CSVFormatDialog.cpp:366
void columnPurposeChanged(int purpose)
Definition: CSVFormatDialog.cpp:481
void sampleRateChanged(QString)
Definition: CSVFormatDialog.cpp:465
CSVFormatDialog(QWidget *parent, CSVFormat initialFormat, int maxDisplayCols)
Definition: CSVFormatDialog.cpp:38
QList< QComboBox * > m_columnPurposeCombos
Definition: CSVFormatDialog.h:95
void removeStartTimePurpose()
Definition: CSVFormatDialog.cpp:393
std::map< TimingOption, QString > m_timingLabels
Definition: CSVFormatDialog.h:70
void updateFormatFromDialog()
Definition: CSVFormatDialog.cpp:565
Generated by
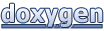