svgui
1.9
|
CSVExportDialog.h
Go to the documentation of this file.
bool shouldIncludeHeader() const
Return true if we should include a header row at the top of the exported file.
Definition: CSVExportDialog.cpp:206
bool shouldConstrainToSelection() const
Return true if we should export the selected time range(s) only.
Definition: CSVExportDialog.cpp:230
bool shouldConstrainToViewHeight() const
Return true if we should constrain the vertical range to the visible area only.
Definition: CSVExportDialog.cpp:224
bool haveView
True if we have a view that provides a vertical scale range, so we may want to offer a choice between...
Definition: CSVExportDialog.h:60
Definition: CSVExportDialog.h:25
bool shouldIncludeTimestamps() const
Return true if we should write a timestamp column.
Definition: CSVExportDialog.cpp:212
bool shouldWriteTimeInFrames() const
Return true if we should use sample frames rather than seconds for the timestamp column (and duration...
Definition: CSVExportDialog.cpp:218
QString getDelimiter() const
Return the column delimiter to use in the exported file.
Definition: CSVExportDialog.cpp:200
bool haveSelection
True if there is a selection current that the user may want to constrain export to.
Definition: CSVExportDialog.h:66
bool isDense
True if the model is a dense type for which timestamps are not written by default.
Definition: CSVExportDialog.h:52
CSVExportDialog(Configuration config, QWidget *parent)
!! todo: remember & re-apply last set of options chosen for this layer type
Definition: CSVExportDialog.cpp:35
Generated by
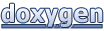