svgui
1.9
|
CSVAudioFormatDialog.h
Go to the documentation of this file.
Definition: CSVAudioFormatDialog.h:27
void updateFormatFromDialog()
Definition: CSVAudioFormatDialog.cpp:195
void sampleRangeChanged(int)
Definition: CSVAudioFormatDialog.cpp:183
QList< QComboBox * > m_columnPurposeCombos
Definition: CSVAudioFormatDialog.h:53
void sampleRateChanged(QString)
Definition: CSVAudioFormatDialog.cpp:175
QComboBox * m_sampleRangeCombo
Definition: CSVAudioFormatDialog.h:51
void columnPurposeChanged(int purpose)
Definition: CSVAudioFormatDialog.cpp:189
QComboBox * m_sampleRateCombo
Definition: CSVAudioFormatDialog.h:50
CSVAudioFormatDialog(QWidget *parent, CSVFormat initialFormat, int maxDisplayCols=5)
Definition: CSVAudioFormatDialog.cpp:37
~CSVAudioFormatDialog()
Definition: CSVAudioFormatDialog.cpp:164
Generated by
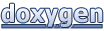