svcore
1.9
|
UnitDatabase.h
Go to the documentation of this file.
Definition: UnitDatabase.h:29
int getUnitId(QString unit, bool registerNew=true)
Return the reference id for a given unit name.
Definition: UnitDatabase.cpp:52
void unitDatabaseChanged()
Generated by
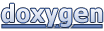