svcore
1.9
|
StringBits.h
Go to the documentation of this file.
static bool isValidUtf8(const std::string &bytes, bool isTruncated)
Return true if the given byte array contains a valid UTF-8 sequence, false if not.
Definition: StringBits.cpp:189
static QStringList split(QString s, QChar separator, bool quoted)
Split a string at the given separator character.
Definition: StringBits.cpp:160
Definition: StringBits.h:45
Definition: StringBits.h:42
Definition: StringBits.h:28
Definition: StringBits.h:43
static QString joinDelimited(QVector< QString > row, QString delimiter)
Join a vector of strings into a single string, with the delimiter as the joining string.
Definition: StringBits.cpp:172
static QStringList splitQuoted(QString s, QChar separator, EscapeMode escapeMode=EscapeAny)
Split a string at the given separator character, allowing quoted sections that contain the separator...
Definition: StringBits.cpp:75
Definition: StringBits.h:44
static double stringToDoubleLocaleFree(QString s, bool *ok=0)
Convert a string to a double using basic "C"-locale syntax, i.e.
Definition: StringBits.cpp:28
Generated by
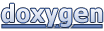