svcore
1.9
|
Serialiser.cpp
Go to the documentation of this file.
~Serialiser()
Release the lock associated with the given id (if taken, rather than cancelled).
Definition: Serialiser.cpp:66
Serialiser(QString id)
Construct a serialiser that takes the lock associated with the given id.
Definition: Serialiser.cpp:27
Definition: Serialiser.h:25
Generated by
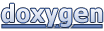