svcore
1.9
|
RDFImporter.h
Go to the documentation of this file.
static QString getKnownExtensions()
Return the file extensions that we have data file readers for, in a format suitable for use with QFil...
Definition: RDFImporter.cpp:91
Definition: ProgressReporter.h:22
static bool isPlausibleDocumentOfAnyKind(QUrl url)
Definition: RDFImporter.cpp:889
Definition: RDFImporter.h:30
Definition: RDFImporter.h:60
void setSampleRate(sv_samplerate_t sampleRate)
Definition: RDFImporter.cpp:107
Definition: RDFImporter.h:61
std::vector< ModelId > getDataModels(ProgressReporter *reporter)
Return a list of models imported from the RDF source.
Definition: RDFImporter.cpp:125
Definition: RDFImporter.cpp:52
Definition: RDFImporter.h:62
RDFImporter(QString url, sv_samplerate_t sampleRate=0)
Definition: RDFImporter.cpp:96
static RDFDocumentType identifyDocumentType(QUrl url)
Definition: RDFImporter.cpp:795
Definition: RDFImporter.h:59
Generated by
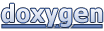