svcore
1.9
|
PluginPathSetter.h
Go to the documentation of this file.
static std::vector< TypeKey > getSupportedKeys()
Definition: PluginPathSetter.cpp:48
static QString getSettingTagFor(TypeKey)
Definition: PluginPathSetter.cpp:145
static void initialiseEnvironmentVariables()
Update *_PATH environment variables from the settings, on application startup.
Definition: PluginPathSetter.cpp:233
static QString getOriginalEnvironmentValue(QString envVariable)
Return the original value observed on startup for the given environment variable, if it is one of the...
Definition: PluginPathSetter.cpp:223
static std::map< QString, QString > m_originalEnvValues
Definition: PluginPathSetter.h:76
static Paths getDefaultPaths()
Return default values of paths only, without any environment variables or user-defined preferences...
Definition: PluginPathSetter.cpp:102
static Paths getPaths()
Return paths arising from user settings + environment variables + defaults as appropriate.
Definition: PluginPathSetter.cpp:155
static Paths m_environmentPaths
Definition: PluginPathSetter.h:75
std::pair< KnownPlugins::PluginType, KnownPlugins::BinaryFormat > TypeKey
Definition: PluginPathSetter.h:30
static void savePathSettings(Paths paths)
Save the given paths to the settings.
Definition: PluginPathSetter.cpp:204
static Paths getEnvironmentPathsUncached(const TypeKeys &keys)
Definition: PluginPathSetter.cpp:78
static Paths getEnvironmentPaths()
Return paths arising from environment variables only, falling back to the defaults, without any user-defined preferences.
Definition: PluginPathSetter.cpp:130
Definition: PluginPathSetter.h:26
Generated by
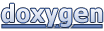