svcore
1.9
|
HelperExecPath.cpp
Go to the documentation of this file.
QString getHelperExecutable(QString basename)
Find a helper executable with the given base name in the bundle directory or installation location...
Definition: HelperExecPath.cpp:51
QStringList getHelperDirPaths()
Return the list of directories searched for helper executables.
Definition: HelperExecPath.cpp:59
QList< HelperExec > search(QString, QStringList &)
Definition: HelperExecPath.cpp:103
QStringList getHelperCandidatePaths(QString basename)
Return the list of executable paths examined in the search for the helper executable with the given b...
Definition: HelperExecPath.cpp:95
QList< HelperExec > getHelperExecutables(QString basename)
Find all helper executables with the given base name in the bundle directory or installation location...
Definition: HelperExecPath.cpp:44
Generated by
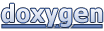