svcore
1.9
|
FileReadThread.h
Go to the documentation of this file.
Definition: FileReadThread.h:39
Definition: FileReadThread.h:29
Definition: Thread.h:24
virtual bool getRequest(int token, Request &request)
Definition: FileReadThread.cpp:170
Generated by
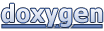