svcore
1.9
|
AudioLevel.h
Go to the documentation of this file.
Definition: AudioLevel.h:44
static double preview_to_multiplier(int level, int levels)
Definition: AudioLevel.cpp:227
Definition: AudioLevel.h:45
static double fader_to_multiplier(int level, int maxLevel, FaderType type)
Definition: AudioLevel.cpp:204
static double multiplier_to_dB(double multiplier)
Definition: AudioLevel.cpp:50
static int multiplier_to_preview(double multiplier, int levels)
Definition: AudioLevel.cpp:220
Definition: AudioLevel.h:42
static int multiplier_to_fader(double multiplier, int maxFaderLevel, FaderType type)
Definition: AudioLevel.cpp:211
Definition: AudioLevel.h:41
Definition: AudioLevel.h:43
AudioLevel converts audio sample levels between various scales:
Definition: AudioLevel.h:34
static int dB_to_fader(double dB, int maxFaderLevel, FaderType type)
Definition: AudioLevel.cpp:150
static double fader_to_dB(int level, int maxLevel, FaderType type)
Definition: AudioLevel.cpp:113
Generated by
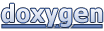