svapp
1.9
|
SVFileReader loads Sonic Visualiser XML files. More...
#include <SVFileReader.h>
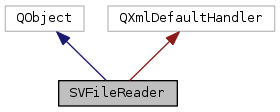
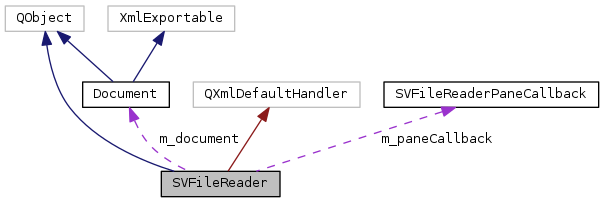
Classes | |
struct | PendingAggregateRec |
Public Types | |
enum | FileType { SVSessionFile, SVLayerFile, UnknownFileType } |
Signals | |
void | modelRegenerationFailed (QString layerName, QString transformName, QString message) |
void | modelRegenerationWarning (QString layerName, QString transformName, QString message) |
Public Member Functions | |
SVFileReader (Document *document, SVFileReaderPaneCallback &callback, QString location="") | |
virtual | ~SVFileReader () |
void | parse (const QString &xmlData) |
void | parse (QXmlInputSource &source) |
bool | isOK () |
QString | getErrorString () const |
void | setCurrentPane (Pane *pane) |
bool | startElement (const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts) override |
bool | characters (const QString &) override |
bool | endElement (const QString &namespaceURI, const QString &localName, const QString &qName) override |
bool | error (const QXmlParseException &exception) override |
bool | fatalError (const QXmlParseException &exception) override |
Static Public Member Functions | |
static FileType | identifyXmlFile (QString path) |
Protected Types | |
typedef XmlExportable::ExportId | ExportId |
Protected Member Functions | |
bool | readWindow (const QXmlAttributes &) |
bool | readModel (const QXmlAttributes &) |
bool | readView (const QXmlAttributes &) |
bool | readLayer (const QXmlAttributes &) |
bool | readDatasetStart (const QXmlAttributes &) |
bool | addBinToDataset (const QXmlAttributes &) |
bool | addPointToDataset (const QXmlAttributes &) |
bool | addRowToDataset (const QXmlAttributes &) |
bool | readRowData (const QString &) |
bool | readDerivation (const QXmlAttributes &) |
bool | readPlayParameters (const QXmlAttributes &) |
bool | readPlugin (const QXmlAttributes &) |
bool | readPluginForTransform (const QXmlAttributes &) |
bool | readPluginForPlayback (const QXmlAttributes &) |
bool | readTransform (const QXmlAttributes &) |
bool | readParameter (const QXmlAttributes &) |
bool | readSelection (const QXmlAttributes &) |
bool | readMeasurement (const QXmlAttributes &) |
void | makeAggregateModels () |
void | addUnaddedModels () |
bool | haveModel (ExportId id) |
Protected Attributes | |
Document * | m_document |
SVFileReaderPaneCallback & | m_paneCallback |
QString | m_location |
Pane * | m_currentPane |
std::map< ExportId, Layer * > | m_layers |
std::map< ExportId, ModelId > | m_models |
std::map< ExportId, Path * > | m_paths |
std::set< ModelId > | m_addedModels |
std::map< ExportId, PendingAggregateRec > | m_pendingAggregates |
std::map< ExportId, ExportId > | m_awaitingDatasets |
ExportId | m_currentDataset |
Layer * | m_currentLayer |
ModelId | m_currentDerivedModel |
ExportId | m_pendingDerivedModel |
std::shared_ptr< PlayParameters > | m_currentPlayParameters |
Transform | m_currentTransform |
ModelId | m_currentTransformSource |
int | m_currentTransformChannel |
bool | m_currentTransformIsNewStyle |
QString | m_datasetSeparator |
bool | m_inRow |
bool | m_inLayer |
bool | m_inView |
bool | m_inData |
bool | m_inSelections |
int | m_rowNumber |
QString | m_errorString |
bool | m_ok |
Detailed Description
SVFileReader loads Sonic Visualiser XML files.
(The SV file format is bzipped XML.)
Some notes about the SV XML format follow. We're very lazy with our XML: there's no schema or DTD, and we depend heavily on elements being in a particular order.
<sv> <data> <!-- The data section contains definitions of both models and visual layers. Layers are considered data in the document; the structure of views that displays the layers is not. --> <!-- id numbers are unique within the data type (i.e. no two models can have the same id, but a model can have the same id as a layer, etc). SV generates its id numbers just for the purpose of cross-referencing within the current file; they don't necessarily have any meaning once the file has been loaded. --> <model id="0" name="..." type="..." ... /> <model id="1" name="..." type="..." ... /> <!-- Models that have data associated with them store it in a neighbouring dataset element. The dataset must follow the model and precede any derivation or layer elements that refer to the model. --> <model id="2" name="..." type="..." dataset="0" ... /> <dataset id="0" type="..."> <point frame="..." value="..." ... /> </dataset> <!-- Where one model is derived from another via a transform, it has an associated derivation element. This must follow both the source and target model elements. The source and model attributes give the source model id and target model id respectively. A model can have both dataset and derivation elements; if it does, dataset must appear first. If the model's data are not stored, but instead the model is to be regenerated completely from the transform when the session is reloaded, then the model should have _only_ a derivation element, and no model element should appear for it at all. --> <derivation type="transform" source="0" model="2" channel="-1"> <transform id="vamp:soname:pluginid:output" ... /> </derivation> <!-- Note that the derivation element just described replaces this earlier formulation, which had more attributes in the derivation element and a plugin element describing plugin parameters and properties. What we actually read and write these days is a horrid composite of the two formats, for backward compatibility reasons. --> <derivation source="0" model="2" transform="vamp:soname:pluginid:output" ...> <plugin id="pluginid" ... /> </derivation> <!-- The playparameters element lists playback settings for a model. --> <playparameters mute="false" pan="0" gain="1" model="1" ... /> <!-- Layer elements. The models must have already been defined. The same model may appear in more than one layer (of more than one type). --> <layer id="1" type="..." name="..." model="0" ... /> <layer id="2" type="..." name="..." model="1" ... /> </data> <display> <!-- The display element contains visual structure for the layers. It's simpler than the data section. --> <!-- Overall preferred window size for this session. (Now deprecated, it wasn't a good idea to try to persist this) --> <window width="..." height="..."/> <!-- List of view elements to stack up. Each one contains a list of layers in stacking order, back to front. --> <view type="pane" ...> <layer id="1"/> <layer id="2"/> </view> <!-- The layer elements just refer to layers defined in the data section, so they don't have to have any attributes other than the id. For sort-of-historical reasons SV actually does repeat the other attributes here, but it doesn't need to. --> <view type="pane" ...> <layer id="2"/> <view> </display> <!-- List of selected regions by audio frame extents. --> <selections> <selection start="..." end="..."/> </selections> </sv>
Definition at line 166 of file SVFileReader.h.
Member Typedef Documentation
|
protected |
Definition at line 247 of file SVFileReader.h.
Member Enumeration Documentation
Enumerator | |
---|---|
SVSessionFile | |
SVLayerFile | |
UnknownFileType |
Definition at line 199 of file SVFileReader.h.
Constructor & Destructor Documentation
SVFileReader::SVFileReader | ( | Document * | document, |
SVFileReaderPaneCallback & | callback, | ||
QString | location = "" |
||
) |
Definition at line 55 of file SVFileReader.cpp.
|
virtual |
Definition at line 101 of file SVFileReader.cpp.
References m_addedModels, m_awaitingDatasets, m_models, and m_paths.
Member Function Documentation
void SVFileReader::parse | ( | const QString & | xmlData | ) |
Definition at line 79 of file SVFileReader.cpp.
void SVFileReader::parse | ( | QXmlInputSource & | source | ) |
Definition at line 87 of file SVFileReader.cpp.
References m_ok.
bool SVFileReader::isOK | ( | ) |
Definition at line 96 of file SVFileReader.cpp.
References m_ok.
|
inline |
Definition at line 180 of file SVFileReader.h.
|
inline |
Definition at line 183 of file SVFileReader.h.
|
override |
Definition at line 137 of file SVFileReader.cpp.
References addBinToDataset(), addPointToDataset(), addRowToDataset(), addUnaddedModels(), m_inData, m_inSelections, m_inView, makeAggregateModels(), readDatasetStart(), readDerivation(), readLayer(), readMeasurement(), readModel(), readParameter(), readPlayParameters(), readPlugin(), readSelection(), readTransform(), readView(), and readWindow().
|
override |
Definition at line 263 of file SVFileReader.cpp.
References m_inRow, m_rowNumber, and readRowData().
|
override |
Definition at line 278 of file SVFileReader.cpp.
References Document::addAlreadyDerivedModel(), Document::addDerivedModel(), addUnaddedModels(), haveModel(), m_addedModels, m_awaitingDatasets, m_currentDataset, m_currentDerivedModel, m_currentPlayParameters, m_currentTransform, m_currentTransformChannel, m_currentTransformSource, m_document, m_inData, m_inLayer, m_inRow, m_inSelections, m_inView, m_models, m_pendingDerivedModel, modelRegenerationFailed(), and modelRegenerationWarning().
|
override |
Definition at line 368 of file SVFileReader.cpp.
References m_errorString.
|
override |
Definition at line 380 of file SVFileReader.cpp.
References m_errorString.
|
static |
Definition at line 1636 of file SVFileReader.cpp.
References SVFileIdentifier::getType(), and SVFileIdentifier::parse().
Referenced by MainWindowBase::openLayer(), and MainWindowBase::openSession().
|
signal |
Referenced by endElement().
|
signal |
Referenced by endElement().
|
protected |
Definition at line 400 of file SVFileReader.cpp.
Referenced by startElement().
|
protected |
Definition at line 489 of file SVFileReader.cpp.
References Document::getMainModel(), haveModel(), m_addedModels, m_awaitingDatasets, m_document, m_location, m_models, m_paths, m_pendingAggregates, READ_MANDATORY, Document::setIncomplete(), and Document::setMainModel().
Referenced by startElement().
|
protected |
Definition at line 831 of file SVFileReader.cpp.
References SVFileReaderPaneCallback::addPane(), m_currentPane, m_paneCallback, and READ_MANDATORY.
Referenced by startElement().
|
protected |
Definition at line 897 of file SVFileReader.cpp.
References Document::addLayerToView(), Document::createLayer(), Document::deleteLayer(), haveModel(), m_currentLayer, m_currentPane, m_document, m_inData, m_inLayer, m_layers, m_models, and Document::setModel().
Referenced by startElement().
|
protected |
Definition at line 1028 of file SVFileReader.cpp.
References haveModel(), m_awaitingDatasets, m_currentDataset, m_datasetSeparator, m_models, m_paths, and READ_MANDATORY.
Referenced by startElement().
|
protected |
Definition at line 1186 of file SVFileReader.cpp.
References haveModel(), m_currentDataset, and m_models.
Referenced by startElement().
|
protected |
Definition at line 1095 of file SVFileReader.cpp.
References haveModel(), m_currentDataset, m_models, m_paths, and READ_MANDATORY.
Referenced by startElement().
|
protected |
Definition at line 1220 of file SVFileReader.cpp.
References m_inRow, and m_rowNumber.
Referenced by startElement().
|
protected |
Definition at line 1240 of file SVFileReader.cpp.
References haveModel(), m_currentDataset, m_datasetSeparator, m_models, and m_rowNumber.
Referenced by characters().
|
protected |
Definition at line 1287 of file SVFileReader.cpp.
References Document::getMainModel(), haveModel(), m_currentDerivedModel, m_currentTransform, m_currentTransformChannel, m_currentTransformIsNewStyle, m_currentTransformSource, m_document, m_models, and m_pendingDerivedModel.
Referenced by startElement().
|
protected |
Definition at line 1380 of file SVFileReader.cpp.
References haveModel(), m_currentPlayParameters, and m_models.
Referenced by startElement().
|
protected |
Definition at line 1435 of file SVFileReader.cpp.
References m_currentPlayParameters, m_pendingDerivedModel, readPluginForPlayback(), and readPluginForTransform().
Referenced by startElement().
|
protected |
Definition at line 1448 of file SVFileReader.cpp.
References m_currentTransform, and m_currentTransformIsNewStyle.
Referenced by readPlugin().
|
protected |
Definition at line 1472 of file SVFileReader.cpp.
References m_currentPlayParameters.
Referenced by readPlugin().
|
protected |
Definition at line 1486 of file SVFileReader.cpp.
References m_currentTransform, and m_pendingDerivedModel.
Referenced by startElement().
|
protected |
Definition at line 1499 of file SVFileReader.cpp.
References m_currentTransform, and m_pendingDerivedModel.
Referenced by startElement().
|
protected |
Definition at line 1520 of file SVFileReader.cpp.
References SVFileReaderPaneCallback::addSelection(), m_paneCallback, and READ_MANDATORY.
Referenced by startElement().
|
protected |
Definition at line 1533 of file SVFileReader.cpp.
References m_currentLayer, and m_inLayer.
Referenced by startElement().
|
protected |
Definition at line 410 of file SVFileReader.cpp.
References SVFileReader::PendingAggregateRec::components, m_models, m_pendingAggregates, and SVFileReader::PendingAggregateRec::name.
Referenced by addUnaddedModels(), and startElement().
|
protected |
Definition at line 467 of file SVFileReader.cpp.
References Document::addNonDerivedModel(), m_addedModels, m_document, m_models, and makeAggregateModels().
Referenced by endElement(), and startElement().
|
inlineprotected |
Definition at line 249 of file SVFileReader.h.
Referenced by addBinToDataset(), addPointToDataset(), endElement(), readDatasetStart(), readDerivation(), readLayer(), readModel(), readPlayParameters(), and readRowData().
Member Data Documentation
|
protected |
Definition at line 259 of file SVFileReader.h.
Referenced by addUnaddedModels(), endElement(), readDerivation(), readLayer(), and readModel().
|
protected |
Definition at line 260 of file SVFileReader.h.
Referenced by readSelection(), and readView().
|
protected |
Definition at line 261 of file SVFileReader.h.
Referenced by readModel().
|
protected |
Definition at line 262 of file SVFileReader.h.
Referenced by readLayer(), and readView().
|
protected |
Definition at line 263 of file SVFileReader.h.
Referenced by readLayer().
|
protected |
Definition at line 264 of file SVFileReader.h.
Referenced by addBinToDataset(), addPointToDataset(), addUnaddedModels(), endElement(), makeAggregateModels(), readDatasetStart(), readDerivation(), readLayer(), readModel(), readPlayParameters(), readRowData(), and ~SVFileReader().
|
protected |
Definition at line 265 of file SVFileReader.h.
Referenced by addPointToDataset(), readDatasetStart(), readModel(), and ~SVFileReader().
|
protected |
Definition at line 266 of file SVFileReader.h.
Referenced by addUnaddedModels(), endElement(), readModel(), and ~SVFileReader().
|
protected |
Definition at line 267 of file SVFileReader.h.
Referenced by makeAggregateModels(), and readModel().
Definition at line 275 of file SVFileReader.h.
Referenced by endElement(), readDatasetStart(), readModel(), and ~SVFileReader().
|
protected |
Definition at line 280 of file SVFileReader.h.
Referenced by addBinToDataset(), addPointToDataset(), endElement(), readDatasetStart(), and readRowData().
|
protected |
Definition at line 282 of file SVFileReader.h.
Referenced by readLayer(), and readMeasurement().
|
protected |
Definition at line 283 of file SVFileReader.h.
Referenced by endElement(), and readDerivation().
|
protected |
Definition at line 284 of file SVFileReader.h.
Referenced by endElement(), readDerivation(), readParameter(), readPlugin(), and readTransform().
|
protected |
Definition at line 285 of file SVFileReader.h.
Referenced by endElement(), readPlayParameters(), readPlugin(), and readPluginForPlayback().
|
protected |
Definition at line 286 of file SVFileReader.h.
Referenced by endElement(), readDerivation(), readParameter(), readPluginForTransform(), and readTransform().
|
protected |
Definition at line 287 of file SVFileReader.h.
Referenced by endElement(), and readDerivation().
|
protected |
Definition at line 288 of file SVFileReader.h.
Referenced by endElement(), and readDerivation().
|
protected |
Definition at line 289 of file SVFileReader.h.
Referenced by readDerivation(), and readPluginForTransform().
|
protected |
Definition at line 290 of file SVFileReader.h.
Referenced by readDatasetStart(), and readRowData().
|
protected |
Definition at line 291 of file SVFileReader.h.
Referenced by addRowToDataset(), characters(), and endElement().
|
protected |
Definition at line 292 of file SVFileReader.h.
Referenced by endElement(), readLayer(), and readMeasurement().
|
protected |
Definition at line 293 of file SVFileReader.h.
Referenced by endElement(), and startElement().
|
protected |
Definition at line 294 of file SVFileReader.h.
Referenced by endElement(), SVFileIdentifier::endElement(), readLayer(), startElement(), and SVFileIdentifier::startElement().
|
protected |
Definition at line 295 of file SVFileReader.h.
Referenced by endElement(), and startElement().
|
protected |
Definition at line 296 of file SVFileReader.h.
Referenced by addRowToDataset(), characters(), and readRowData().
|
protected |
Definition at line 297 of file SVFileReader.h.
Referenced by error(), and fatalError().
|
protected |
Definition at line 298 of file SVFileReader.h.
The documentation for this class was generated from the following files:
Generated by
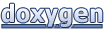