svapp
1.9
|
Document.h
Go to the documentation of this file.
Definition: Document.h:136
void setMainModel(ModelId)
Set the main model (the source for playback sample rate, etc) to the given wave file model...
Definition: Document.cpp:421
std::vector< ModelId > getTransformInputModels()
Definition: Document.cpp:1083
void setChannel(Layer *, int)
Set the given layer to use the given channel of its model (-1 means all available channels)...
Definition: Document.cpp:987
void writeBackwardCompatibleDerivation(QTextStream &, QString, ModelId, const ModelRecord &) const
Definition: Document.cpp:1568
void writePlaceholderMainModel(QTextStream &, QString) const
Definition: Document.cpp:1557
void mainModelChanged(ModelId)
void cancelAsyncLayerCreation(LayerCreationAsyncHandle handle)
Indicate that the async layer creation task associated with the given handle should be cancelled...
Definition: Document.cpp:362
void activity(QString)
virtual ~LayerCreationHandler()
Definition: Document.h:138
void layerAboutToBeDeleted(Layer *)
void layerRemoved(Layer *)
LayerCreationAsyncHandle createDerivedLayersAsync(const Transforms &, const ModelTransformer::Input &, LayerCreationHandler *handler)
Create suitable layers for the given transforms, which must be identical apart from the output (i...
Definition: Document.cpp:324
void modelRegenerationWarning(QString layerName, QString transformName, QString message)
std::map< Layer *, std::set< View * > > LayerViewMap
Definition: Document.h:434
Layer * createMainModelLayer(LayerFactory::LayerType)
Create and return a new layer of the given type, associated with the current main model (if appropria...
Definition: Document.cpp:140
void modelGenerationFailed(QString transformName, QString message)
Layer * createLayer(LayerFactory::LayerType)
Create and return a new layer of the given type, associated with no model.
Definition: Document.cpp:118
void performDeferredAlignment(ModelId)
Definition: Document.cpp:1194
void layerAdded(Layer *)
void realignModels()
Re-generate alignments for all appropriate models against the main model.
Definition: Document.cpp:1210
Document()
!! still need to handle command history, documentRestored/documentModified
Definition: Document.cpp:48
void modelAdded(ModelId)
ModelId getMainModel()
Get the main model (the source for playback sample rate, etc).
Definition: Document.h:195
void alignModels()
Generate alignments for all appropriate models against the main model.
Definition: Document.cpp:1201
void addAdditionalModel(ModelId)
Add an extra derived model (returned at the end of processing a transform).
Definition: Document.cpp:697
void toXmlAsTemplate(QTextStream &, QString indent, QString extraAttributes) const
Definition: Document.cpp:1351
void modelRegenerationFailed(QString layerName, QString transformName, QString message)
void removeLayerFromView(View *, Layer *)
Remove the given layer from the given view.
Definition: Document.cpp:1018
void alignModel(ModelId, bool forceRecalculate=false)
If model is suitable for alignment, align it against the main model and store the alignment in the mo...
Definition: Document.cpp:1124
ModelId m_mainModel
The model that provides the underlying sample rate, etc.
Definition: Document.h:363
Layer * createEmptyLayer(LayerFactory::LayerType)
Create and return a new layer of the given type, with an appropriate empty model. ...
Definition: Document.cpp:189
std::vector< Layer * > createLayersForDerivedModels(std::vector< ModelId >, QStringList names)
Definition: Document.cpp:369
QString getUniqueLayerName(QString candidate)
Definition: Document.cpp:1061
void layerInAView(Layer *, bool)
void toXml(QTextStream &, QString indent, QString extraAttributes) const override
Definition: Document.cpp:1345
bool isKnownModel(ModelId) const
Return true if the model id is known to be the main model or one of the other existing models that ca...
Definition: Document.cpp:1108
void alignmentFailed(ModelId, QString message)
Layer * createDerivedLayer(LayerFactory::LayerType, TransformId)
Create and return a new layer of the given type, associated with the given transform name...
Definition: Document.cpp:212
A Sonic Visualiser document consists of a set of data models, and also the visualisation layers used ...
Definition: Document.h:71
Definition: Document.h:365
bool isIncomplete() const
Return true if any external files (most obviously audio) failed to be found on load, so that the document is incomplete compared to its saved description.
Definition: Document.h:307
void modelGenerationWarning(QString transformName, QString message)
void addLayerToView(View *, Layer *)
Add the given layer to the given view.
Definition: Document.cpp:993
Definition: Document.h:397
Definition: Document.cpp:271
void setAutoAlignment(bool on)
Specify whether models added via addImportedModel should be automatically aligned against the main mo...
Definition: Document.h:287
void setModel(Layer *, ModelId)
Associate the given model with the given layer.
Definition: Document.cpp:951
void removeFromLayerViewMap(Layer *, View *)
Definition: Document.cpp:1043
std::vector< Layer * > createDerivedLayers(const Transforms &, const ModelTransformer::Input &)
Create and return suitable layers for the given transforms, which must be identical apart from the ou...
Definition: Document.cpp:241
virtual void layersCreated(LayerCreationAsyncHandle handle, std::vector< Layer * > primary, std::vector< Layer * > additional)=0
The primary layers are those corresponding 1-1 to the input models, listed in the same order as the i...
void alignmentComplete(ModelId)
Definition: Document.h:415
void addAlreadyDerivedModel(const Transform &transform, const ModelTransformer::Input &input, ModelId outputModelToAdd)
Add a derived model associated with the given transform.
Definition: Document.cpp:604
void deleteLayer(Layer *, bool force=false)
Delete the given layer, and also its associated model if no longer used by any other layer...
Definition: Document.cpp:889
ModelId addDerivedModel(const Transform &transform, const ModelTransformer::Input &input, QString &returnedMessage)
Add a derived model associated with the given transform, running the transform and returning the resu...
Definition: Document.cpp:733
Layer * createImportedLayer(ModelId)
Create and return a new layer associated with the given model, and register the model as an imported ...
Definition: Document.cpp:151
std::vector< ModelId > addDerivedModels(const Transforms &transforms, const ModelTransformer::Input &input, QString &returnedMessage, AdditionalModelConverter *)
Definition: Document.cpp:756
Generated by
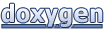